diff --git a/docs/_config.yml b/docs/_config.yml
index 4dc5eee07d..540e3ad8ae 100644
--- a/docs/_config.yml
+++ b/docs/_config.yml
@@ -3,7 +3,7 @@ repository: pmd/pmd
pmd:
version: 7.0.0-SNAPSHOT
previous_version: 6.55.0
- date: ??-????-2023
+ date: 27-May-2023
release_type: major
# release types: major, minor, bugfix
diff --git a/docs/pages/pmd/devdocs/how_pmd_works.md b/docs/pages/pmd/devdocs/how_pmd_works.md
index 1ac3f99929..f91b76757d 100644
--- a/docs/pages/pmd/devdocs/how_pmd_works.md
+++ b/docs/pages/pmd/devdocs/how_pmd_works.md
@@ -9,10 +9,7 @@ author: Tom Copeland, Andreas Dangel
PMD 7.0.0 is finally almost ready. In order to gather feedback, we are going to ship a couple of release candidates. +These are officially available on GitHub and Maven Central and can be used as usual (e.g. as a dependency). +We encourage you to try out the new features, but keep in mind that we may introduce API breaking changes between +the release candidates. It should be stable enough if you don't use custom rules.
+ +We have still some tasks planned for the next release candidates. +You can see the progress in PMD 7 Tracking Issue #3898.
+ +If you find any problem or difficulty while updating from PMD 6, please provide feedback via our +issue tracker. That way we can improve the experience +for all.
+AntlrTokenizer
and JavaCCTokenizer
from
+ `internal` package into package net.sourceforge.pmd.cpd.impl
. These two classes are part of the API and
+ are base classes for CPD language implementations.
+* `AntlrBaseRule` is gone in favor of AbstractVisitorRule
.
+* The classes KotlinInnerNode
and SwiftInnerNode
+ are package-private now.
+* The parameter order of addSourceFile
has been swapped
+ in order to have the same meaning as in 6.55.0. That will make it easier if you upgrade from 6.55.0 to 7.0.0.
+ However, that means, that you need to change these method calls if you have migrated to 7.0.0-rc1 already.
+
+#### Updated PMD Designer
+This PMD release ships a new version of the pmd-designer.
+For the changes, see [PMD Designer Changelog](https://github.com/pmd/pmd-designer/releases/tag/7.0.0-rc1).
+
+#### Language Related Changes
+* New: CPD support for TypeScript
+* New: CPD support for Julia
+
+#### Rule Changes
+* [`ImmutableField`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_java_design.html#immutablefield): the property `ignoredAnnotations` has been removed. The property was
+ deprecated since PMD 6.52.0.
+* [`SwitchDensity`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_java_design.html#switchdensity): the type of the property `minimum` has been changed from decimal to integer
+ for consistency with other statistical rules.
+
+#### Fixed Issues
+* cli
+ * [#4482](https://github.com/pmd/pmd/issues/4482): \[cli] pmd.bat can only be executed once
+ * [#4484](https://github.com/pmd/pmd/issues/4484): \[cli] ast-dump with no properties produce an NPE
+* core
+ * [#2500](https://github.com/pmd/pmd/issues/2500): \[core] Clarify API for ANTLR based languages
+* doc
+ * [#2501](https://github.com/pmd/pmd/issues/2501): \[doc] Verify ANTLR Documentation
+ * [#4438](https://github.com/pmd/pmd/issues/4438): \[doc] Documentation links in VS Code are outdated
+* java-codestyle
+ * [#4273](https://github.com/pmd/pmd/issues/4273): \[java] CommentDefaultAccessModifier ignoredAnnotations should include "org.junit.jupiter.api.extension.RegisterExtension" by default
+ * [#4487](https://github.com/pmd/pmd/issues/4487): \[java] UnnecessaryConstructor: false-positive with @Inject and @Autowired
+* java-design
+ * [#4254](https://github.com/pmd/pmd/issues/4254): \[java] ImmutableField - false positive with Lombok @Setter
+ * [#4477](https://github.com/pmd/pmd/issues/4477): \[java] SignatureDeclareThrowsException: false-positive with TestNG annotations
+ * [#4490](https://github.com/pmd/pmd/issues/4490): \[java] ImmutableField - false negative with Lombok @Getter
+* java-errorprone
+ * [#4449](https://github.com/pmd/pmd/issues/4449): \[java] AvoidAccessibilityAlteration: Possible false positive in AvoidAccessibilityAlteration rule when using Lambda expression
+ * [#4493](https://github.com/pmd/pmd/issues/4493): \[java] MissingStaticMethodInNonInstantiatableClass: false-positive about @Inject
+ * [#4505](https://github.com/pmd/pmd/issues/4505): \[java] ImplicitSwitchFallThrough NPE in PMD 7.0.0-rc1
+* java-multithreading
+ * [#4483](https://github.com/pmd/pmd/issues/4483): \[java] NonThreadSafeSingleton false positive with double-checked locking
+* miscellaneous
+ * [#4462](https://github.com/pmd/pmd/issues/4462): Provide Software Bill of Materials (SBOM)
+
+#### External contributions
+* [#4402](https://github.com/pmd/pmd/pull/4402): \[javascript] CPD: add support for Typescript using antlr4 grammar - [Paul Guyot](https://github.com/pguyot) (@pguyot)
+* [#4403](https://github.com/pmd/pmd/pull/4403): \[julia] CPD: Add support for Julia code duplication - [Wener](https://github.com/wener-tiobe) (@wener-tiobe)
+* [#4444](https://github.com/pmd/pmd/pull/4444): \[java] CommentDefaultAccessModifier - ignore org.junit.jupiter.api.extension.RegisterExtension by default - [Nirvik Patel](https://github.com/nirvikpatel) (@nirvikpatel)
+* [#4450](https://github.com/pmd/pmd/pull/4450): \[java] Fix #4449 AvoidAccessibilityAlteration: Correctly handle Lambda expressions in PrivilegedAction scenarios - [Seren](https://github.com/mohui1999) (@mohui1999)
+* [#4452](https://github.com/pmd/pmd/pull/4452): \[doc] Update PMD_APEX_ROOT_DIRECTORY documentation reference - [nwcm](https://github.com/nwcm) (@nwcm)
+* [#4474](https://github.com/pmd/pmd/pull/4474): \[java] ImmutableField: False positive with lombok (fixes #4254) - [Pim van der Loos](https://github.com/PimvanderLoos) (@PimvanderLoos)
+* [#4488](https://github.com/pmd/pmd/pull/4488): \[java] Fix #4477: A false-positive about SignatureDeclareThrowsException - [AnnaDev](https://github.com/LynnBroe) (@LynnBroe)
+* [#4494](https://github.com/pmd/pmd/pull/4494): \[java] Fix #4487: A false-positive about UnnecessaryConstructor and @Inject and @Autowired - [AnnaDev](https://github.com/LynnBroe) (@LynnBroe)
+* [#4495](https://github.com/pmd/pmd/pull/4495): \[java] Fix #4493: false-positive about MissingStaticMethodInNonInstantiatableClass and @Inject - [AnnaDev](https://github.com/LynnBroe) (@LynnBroe)
+* [#4520](https://github.com/pmd/pmd/pull/4520): \[doc] Fix typo: missing closing quotation mark after CPD-END - [João Dinis Ferreira](https://github.com/joaodinissf) (@joaodinissf)
+
+### 🚀 Major Features and Enhancements
+
+#### New official logo
+
+The new official logo of PMD:
+
+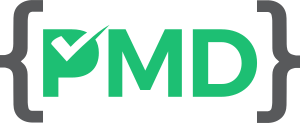
+
+#### Revamped Java module
+
+* Java grammar substantially refactored - more correct regarding the Java Language Specification (JLS)
+* Built-in rules have been upgraded for the changed AST
+* Rewritten type resolution framework and symbol table correctly implements the JLS
+* AST exposes more semantic information (method calls, field accesses)
+
+For more information, see the [Detailed Release Notes for PMD 7](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_release_notes_pmd7.html).
+
+Contributors: [Clément Fournier](https://github.com/oowekyala) (@oowekyala),
+[Andreas Dangel](https://github.com/adangel) (@adangel),
+[Juan Martín Sotuyo Dodero](https://github.com/jsotuyod) (@jsotuyod)
+
+#### Revamped Command Line Interface
+
+* unified and consistent Command Line Interface for both Linux/Unix and Windows across our different utilities
+* single script `pmd` (`pmd.bat` for Windows) to launch the different utilities:
+ * `pmd check` to run PMD rules and analyze a project
+ * `pmd cpd` to run CPD (copy paste detector)
+ * `pmd designer` to run the PMD Rule Designer
+* progress bar support for `pmd check`
+* shell completion
+
+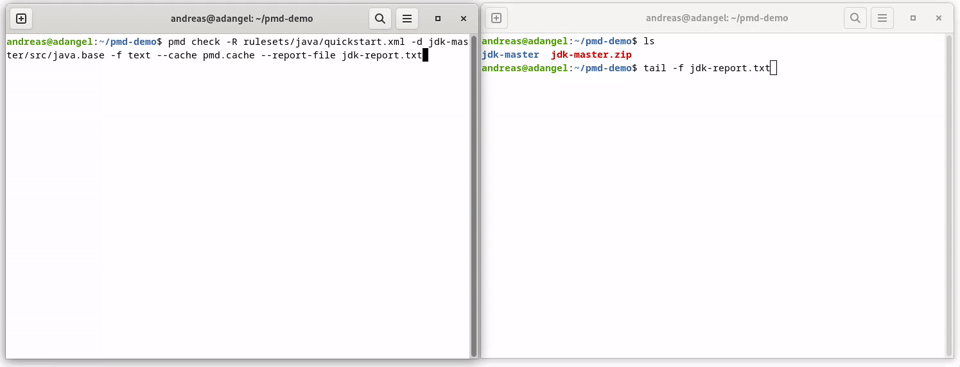
+
+For more information, see the [Detailed Release Notes for PMD 7](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_release_notes_pmd7.html).
+
+Contributors: [Juan Martín Sotuyo Dodero](https://github.com/jsotuyod) (@jsotuyod)
+
+#### Full Antlr support
+
+* [Antlr](https://www.antlr.org/) based grammars can now be used to build full-fledged PMD rules.
+* Previously, Antlr grammar could only be used for CPD
+* New supported languages: Swift and Kotlin
+
+For more information, see the [Detailed Release Notes for PMD 7](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_release_notes_pmd7.html).
+
+Contributors: [Lucas Soncini](https://github.com/lsoncini) (@lsoncini),
+[Matías Fraga](https://github.com/matifraga) (@matifraga),
+[Tomás De Lucca](https://github.com/tomidelucca) (@tomidelucca)
+
+#### Updated PMD Designer
+
+This PMD release ships a new version of the pmd-designer.
+For the changes, see [PMD Designer Changelog](https://github.com/pmd/pmd-designer/releases/tag/7.0.0-rc1).
+
+### 🎉 Language Related Changes
+
+Note that this is just a concise listing of the highlight.
+For more information on the languages, see the [Detailed Release Notes for PMD 7](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_release_notes_pmd7.html).
+
+#### New: Swift support
+
+* use PMD to analyze Swift code with PMD rules
+* initially 4 built-in rules
+
+Contributors: [Lucas Soncini](https://github.com/lsoncini) (@lsoncini),
+[Matías Fraga](https://github.com/matifraga) (@matifraga),
+[Tomás De Lucca](https://github.com/tomidelucca) (@tomidelucca)
+
+#### New: Kotlin support (experimental)
+
+* use PMD to analyze Kotlin code with PMD rules
+* Support for Kotlin 1.8 grammar
+* initially 2 built-in rules
+
+#### New: CPD support for TypeScript
+
+Thanks to a contribution, CPD now supports the TypeScript language. It is shipped
+with the rest of the JavaScript support in the module `pmd-javascript`.
+
+Contributors: [Paul Guyot](https://github.com/pguyot) (@pguyot)
+
+#### New: CPD support for Julia
+
+Thanks to a contribution, CPD now supports the Julia language. It is shipped
+in the new module `pmd-julia`.
+
+Contributors: [Wener](https://github.com/wener-tiobe) (@wener-tiobe)
+
+#### Changed: JavaScript support
+
+* latest version supports ES6 and also some new constructs (see [Rhino](https://github.com/mozilla/rhino)])
+* comments are retained
+
+#### Changed: Language versions
+
+* more predefined language versions for each supported language
+* can be used to limit rule execution for specific versions only with `minimumLanguageVersion` and
+ `maximumLanguageVersion` attributes.
+
+### 🌟 New and changed rules
+
+#### New Rules
+
+**Apex**
+* [`UnusedMethod`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_apex_design.html#unusedmethod) finds unused methods in your code.
+
+**Java**
+* [`UnnecessaryBoxing`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_java_codestyle.html#unnecessaryboxing) reports boxing and unboxing conversions that may be made implicit.
+
+**Kotlin**
+* [`FunctionNameTooShort`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_kotlin_bestpractices.html#functionnametooshort)
+* [`OverrideBothEqualsAndHashcode`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_kotlin_errorprone.html#overridebothequalsandhashcode)
+
+**Swift**
+* [`ProhibitedInterfaceBuilder`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_swift_bestpractices.html#prohibitedinterfacebuilder)
+* [`UnavailableFunction`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_swift_bestpractices.html#unavailablefunction)
+* [`ForceCast`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_swift_errorprone.html#forcecast)
+* [`ForceTry`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_swift_errorprone.html#forcetry)
+
+#### Changed Rules
+
+**General changes**
+
+* All statistical rules (like ExcessiveClassLength, ExcessiveParameterList) have been simplified and unified.
+ The properties `topscore` and `sigma` have been removed. The property `minimum` is still there, however the type is not
+ a decimal number anymore but has been changed to an integer. This affects rules in the languages Apex, Java, PLSQL
+ and Velocity Template Language (vm):
+ * Apex: [`ExcessiveClassLength`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_apex_design.html#excessiveclasslength), [`ExcessiveParameterList`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_apex_design.html#excessiveparameterlist),
+ [`ExcessivePublicCount`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_apex_design.html#excessivepubliccount), [`NcssConstructorCount`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_apex_design.html#ncssconstructorcount),
+ [`NcssMethodCount`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_apex_design.html#ncssmethodcount), [`NcssTypeCount`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_apex_design.html#ncsstypecount)
+ * Java: [`ExcessiveImports`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_java_design.html#excessiveimports), [`ExcessiveParameterList`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_java_design.html#excessiveparameterlist),
+ [`ExcessivePublicCount`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_java_design.html#excessivepubliccount), [`SwitchDensity`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_java_design.html#switchdensity)
+ * PLSQL: [`ExcessiveMethodLength`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_plsql_design.html#excessivemethodlength), [`ExcessiveObjectLength`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_plsql_design.html#excessiveobjectlength),
+ [`ExcessivePackageBodyLength`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_plsql_design.html#excessivepackagebodylength), [`ExcessivePackageSpecificationLength`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_plsql_design.html#excessivepackagespecificationlength),
+ [`ExcessiveParameterList`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_plsql_design.html#excessiveparameterlist), [`ExcessiveTypeLength`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_plsql_design.html#excessivetypelength),
+ [`NcssMethodCount`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_plsql_design.html#ncssmethodcount), [`NcssObjectCount`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_plsql_design.html#ncssobjectcount),
+ [`NPathComplexity`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_plsql_design.html#npathcomplexity)
+ * VM: [`ExcessiveTemplateLength`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_vm_design.html#excessivetemplatelength)
+
+* The general property `violationSuppressXPath` which is available for all rules to
+ [suppress warnings](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_userdocs_suppressing_warnings.html) now uses XPath version 3.1 by default.
+ This version of the XPath language is mostly identical to XPath 2.0. In PMD 6, XPath 1.0 has been used.
+ If you upgrade from PMD 6, you need to verify your `violationSuppressXPath` properties.
+
+**Apex General changes**
+
+* The properties `cc_categories`, `cc_remediation_points_multiplier`, `cc_block_highlighting` have been removed
+ from all rules. These properties have been deprecated since PMD 6.13.0.
+ See [issue #1648](https://github.com/pmd/pmd/issues/1648) for more details.
+
+**Java General changes**
+
+* Violations reported on methods or classes previously reported the line range of the entire method
+ or class. With PMD 7.0.0, the reported location is now just the identifier of the method or class.
+ This affects various rules, e.g. [`CognitiveComplexity`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_java_design.html#cognitivecomplexity).
+
+ The report location is controlled by the overrides of the method getReportLocation
+ in different node types.
+
+ See [issue #4439](https://github.com/pmd/pmd/issues/4439) and [issue #730](https://github.com/pmd/pmd/issues/730)
+ for more details.
+
+**Java Best Practices**
+
+* [`ArrayIsStoredDirectly`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_java_bestpractices.html#arrayisstoreddirectly): Violations are now reported on the assignment and not
+ anymore on the formal parameter. The reported line numbers will probably move.
+* [`AvoidReassigningLoopVariables`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_java_bestpractices.html#avoidreassigningloopvariables): This rule might not report anymore all
+ reassignments of the control variable in for-loops when the property `forReassign` is set to `skip`.
+ See [issue #4500](https://github.com/pmd/pmd/issues/4500) for more details.
+* [`LooseCoupling`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_java_bestpractices.html#loosecoupling): The rule has a new property to allow some types to be coupled
+ to (`allowedTypes`).
+* [`UnusedLocalVariable`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_java_bestpractices.html#unusedlocalvariable): This rule has some important false-negatives fixed
+ and finds many more cases now. For details see issues [#2130](https://github.com/pmd/pmd/issues/2130),
+ [#4516](https://github.com/pmd/pmd/issues/4516), and [#4517](https://github.com/pmd/pmd/issues/4517).
+
+**Java Codestyle**
+
+* [`MethodNamingConventions`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_java_codestyle.html#methodnamingconventions): The property `checkNativeMethods` has been removed. The
+ property was deprecated since PMD 6.3.0. Use the property `nativePattern` to control whether native methods
+ should be considered or not.
+* [`ShortVariable`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_java_codestyle.html#shortvariable): This rule now also reports short enum constant names.
+* [`UseDiamondOperator`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_java_codestyle.html#usediamondoperator): The property `java7Compatibility` has been removed. The rule now
+ handles Java 7 properly without a property.
+* [`UnnecessaryFullyQualifiedName`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_java_codestyle.html#unnecessaryfullyqualifiedname): The rule has two new properties,
+ to selectively disable reporting on static field and method qualifiers. The rule also has been improved
+ to be more precise.
+* [`UselessParentheses`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_java_codestyle.html#uselessparentheses): The rule has two new properties which control how strict
+ the rule should be applied. With `ignoreClarifying` (default: true) parentheses that are strictly speaking
+ not necessary are allowed, if they separate expressions of different precedence.
+ The other property `ignoreBalancing` (default: true) is similar, in that it allows parentheses that help
+ reading and understanding the expressions.
+
+**Java Design**
+
+* [`CyclomaticComplexity`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_java_design.html#cyclomaticcomplexity): The property `reportLevel` has been removed. The property was
+ deprecated since PMD 6.0.0. The report level can now be configured separated for classes and methods using
+ `classReportLevel` and `methodReportLevel` instead.
+* [`ImmutableField`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_java_design.html#immutablefield): The property `ignoredAnnotations` has been removed. The property was
+ deprecated since PMD 6.52.0.
+* [`LawOfDemeter`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_java_design.html#lawofdemeter): The rule has a new property `trustRadius`. This defines the maximum degree
+ of trusted data. The default of 1 is the most restrictive.
+* [`NPathComplexity`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_java_design.html#npathcomplexity): The property `minimum` has been removed. It was deprecated since PMD 6.0.0.
+ Use the property `reportLevel` instead.
+* [`SingularField`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_java_design.html#singularfield): The properties `checkInnerClasses` and `disallowNotAssignment` have been removed.
+ The rule is now more precise and will check these cases properly.
+* [`UseUtilityClass`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_java_design.html#useutilityclass): The property `ignoredAnnotations` has been removed.
+
+**Java Documentation**
+
+* [`CommentContent`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_java_documentation.html#commentcontent): The properties `caseSensitive` and `disallowedTerms` are removed. The
+ new property `forbiddenRegex` can be used now to define the disallowed terms with a single regular
+ expression.
+* [`CommentRequired`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_java_documentation.html#commentrequired):
+ * Overridden methods are now detected even without the `@Override`
+ annotation. This is relevant for the property `methodWithOverrideCommentRequirement`.
+ See also [pull request #3757](https://github.com/pmd/pmd/pull/3757).
+ * Elements in annotation types are now detected as well. This might lead to an increased number of violations
+ for missing public method comments.
+* [`CommentSize`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_java_documentation.html#commentsize): When determining the line-length of a comment, the leading comment
+ prefix markers (e.g. `*` or `//`) are ignored and don't add up to the line-length.
+ See also [pull request #4369](https://github.com/pmd/pmd/pull/4369).
+
+**Java Error Prone**
+
+* [`AvoidDuplicateLiterals`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_java_errorprone.html#avoidduplicateliterals): The property `exceptionfile` has been removed. The property was
+ deprecated since PMD 6.10.0. Use the property `exceptionList` instead.
+* [`DontImportSun`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_java_errorprone.html#dontimportsun): `sun.misc.Signal` is not special-cased anymore.
+* [`EmptyCatchBlock`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_java_errorprone.html#emptycatchblock): `CloneNotSupportedException` and `InterruptedException` are not
+ special-cased anymore. Rename the exception parameter to `ignored` to ignore them.
+* [`ImplicitSwitchFallThrough`](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_rules_java_errorprone.html#implicitswitchfallthrough): Violations are now reported on the case statements
+ rather than on the switch statements. This is more accurate but might result in more violations now.
+
+#### Removed Rules
+
+Many rules, that were previously deprecated have been finally removed.
+See [Detailed Release Notes for PMD 7](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_release_notes_pmd7.html) for the complete list.
+
+### 🚨 API
+
+The API of PMD has been growing over the years and needed some cleanup. The goal is, to
+have a clear separation between a well-defined API and the implementation, which is internal.
+This should help us in future development.
+
+Also, there are some improvement and changes in different areas. For the detailed description
+of the changes listed here, see [Detailed Release Notes for PMD 7](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_release_notes_pmd7.html).
+
+* Miscellaneous smaller changes and cleanups
+* XPath 3.1 support for XPath-based rules
+* Node stream API for AST traversal
+* Metrics framework
+* Testing framework
+* Language Lifecycle and Language Properties
+
+### 💥 Compatibility and migration notes
+See [Detailed Release Notes for PMD 7](https://docs.pmd-code.org/pmd-doc-7.0.0-rc2/pmd_release_notes_pmd7.html).
+
+### 🐛 Fixed Issues
+
+* miscellaneous
+ * [#881](https://github.com/pmd/pmd/issues/881): \[all] Breaking API changes for 7.0.0
+ * [#896](https://github.com/pmd/pmd/issues/896): \[all] Use slf4j
+ * [#1431](https://github.com/pmd/pmd/pull/1431): \[ui] Remove old GUI applications (designerold, bgastviewer)
+ * [#1451](https://github.com/pmd/pmd/issues/1451): \[core] RulesetFactoryCompatibility stores the whole ruleset file in memory as a string
+ * [#2496](https://github.com/pmd/pmd/issues/2496): Update PMD 7 Logo on landing page
+ * [#2497](https://github.com/pmd/pmd/issues/2497): PMD 7 Logo page
+ * [#2498](https://github.com/pmd/pmd/issues/2498): Update PMD 7 Logo in documentation
+ * [#3797](https://github.com/pmd/pmd/issues/3797): \[all] Use JUnit5
+ * [#4462](https://github.com/pmd/pmd/issues/4462): Provide Software Bill of Materials (SBOM)
+* ant
+ * [#4080](https://github.com/pmd/pmd/issues/4080): \[ant] Split off Ant integration into a new submodule
+* core
+ * [#880](https://github.com/pmd/pmd/issues/880): \[core] Make visitors generic
+ * [#1622](https://github.com/pmd/pmd/pull/1622): \[core] NodeStream API
+ * [#1687](https://github.com/pmd/pmd/issues/1687): \[core] Deprecate and Remove XPath 1.0 support
+ * [#1785](https://github.com/pmd/pmd/issues/1785): \[core] Allow abstract node types to be valid rulechain visits
+ * [#1825](https://github.com/pmd/pmd/pull/1825): \[core] Support NoAttribute for XPath
+ * [#2038](https://github.com/pmd/pmd/issues/2038): \[core] Remove DCD
+ * [#2218](https://github.com/pmd/pmd/issues/2218): \[core] `isFindBoundary` should not be an attribute
+ * [#2234](https://github.com/pmd/pmd/issues/2234): \[core] Consolidate PMD CLI into a single command
+ * [#2239](https://github.com/pmd/pmd/issues/2239): \[core] Merging Javacc build scripts
+ * [#2500](https://github.com/pmd/pmd/issues/2500): \[core] Clarify API for ANTLR based languages
+ * [#2518](https://github.com/pmd/pmd/issues/2518): \[core] Language properties
+ * [#2602](https://github.com/pmd/pmd/issues/2602): \[core] Remove ParserOptions
+ * [#2614](https://github.com/pmd/pmd/pull/2614): \[core] Upgrade Saxon, add XPath 3.1, remove Jaxen
+ * [#2696](https://github.com/pmd/pmd/pull/2696): \[core] Remove DFA
+ * [#2821](https://github.com/pmd/pmd/issues/2821): \[core] Rule processing error filenames are missing paths
+ * [#2873](https://github.com/pmd/pmd/issues/2873): \[core] Utility classes in pmd 7
+ * [#2885](https://github.com/pmd/pmd/issues/2885): \[core] Error recovery mode
+ * [#3203](https://github.com/pmd/pmd/issues/3203): \[core] Replace RuleViolationFactory implementations with ViolationDecorator
+ * [#3692](https://github.com/pmd/pmd/pull/3692): \[core] Analysis listeners
+ * [#3782](https://github.com/pmd/pmd/issues/3782): \[core] Language lifecycle
+ * [#3815](https://github.com/pmd/pmd/issues/3815): \[core] Update Saxon HE to 10.7
+ * [#3893](https://github.com/pmd/pmd/pull/3893): \[core] Text documents
+ * [#3902](https://github.com/pmd/pmd/issues/3902): \[core] Violation decorators
+ * [#3918](https://github.com/pmd/pmd/issues/3918): \[core] Make LanguageRegistry non static
+ * [#3922](https://github.com/pmd/pmd/pull/3922): \[core] Better error reporting for the ruleset parser
+ * [#4035](https://github.com/pmd/pmd/issues/4035): \[core] ConcurrentModificationException in DefaultRuleViolationFactory
+ * [#4120](https://github.com/pmd/pmd/issues/4120): \[core] Explicitly name all language versions
+ * [#4353](https://github.com/pmd/pmd/pull/4353): \[core] Micro optimizations for Node API
+ * [#4365](https://github.com/pmd/pmd/pull/4365): \[core] Improve benchmarking
+ * [#4420](https://github.com/pmd/pmd/pull/4420): \[core] Remove PMD.EOL
+* cli
+ * [#2234](https://github.com/pmd/pmd/issues/2234): \[core] Consolidate PMD CLI into a single command
+ * [#3828](https://github.com/pmd/pmd/issues/3828): \[core] Progress reporting
+ * [#4079](https://github.com/pmd/pmd/issues/4079): \[cli] Split off CLI implementation into a pmd-cli submodule
+ * [#4482](https://github.com/pmd/pmd/issues/4482): \[cli] pmd.bat can only be executed once
+ * [#4484](https://github.com/pmd/pmd/issues/4484): \[cli] ast-dump with no properties produce an NPE
+* doc
+ * [#2501](https://github.com/pmd/pmd/issues/2501): \[doc] Verify ANTLR Documentation
+ * [#4438](https://github.com/pmd/pmd/issues/4438): \[doc] Documentation links in VS Code are outdated
+* testing
+ * [#2435](https://github.com/pmd/pmd/issues/2435): \[test] Remove duplicated Dummy language module
+ * [#4234](https://github.com/pmd/pmd/issues/4234): \[test] Tests that change the logging level do not work
+
+Language specific fixes:
+
+* apex
+ * [#1937](https://github.com/pmd/pmd/issues/1937): \[apex] Apex should only have a single RootNode
+ * [#1648](https://github.com/pmd/pmd/issues/1648): \[apex,vf] Remove CodeClimate dependency
+ * [#1750](https://github.com/pmd/pmd/pull/1750): \[apex] Remove apex statistical rules
+ * [#2836](https://github.com/pmd/pmd/pull/2836): \[apex] Remove Apex ProjectMirror
+ * [#4427](https://github.com/pmd/pmd/issues/4427): \[apex] ApexBadCrypto test failing to detect inline code
+* apex-design
+ * [#2667](https://github.com/pmd/pmd/issues/2667): \[apex] Integrate nawforce/ApexLink to build robust Unused rule
+ * [#4509](https://github.com/pmd/pmd/issues/4509): \[apex] ExcessivePublicCount doesn't consider inner classes correctly
+* java
+ * [#520](https://github.com/pmd/pmd/issues/520): \[java] Allow `@SuppressWarnings` with constants instead of literals
+ * [#864](https://github.com/pmd/pmd/issues/864): \[java] Similar/duplicated implementations for determining FQCN
+ * [#905](https://github.com/pmd/pmd/issues/905): \[java] Add new node for anonymous class declaration
+ * [#910](https://github.com/pmd/pmd/issues/910): \[java] AST inconsistency between primitive and reference type arrays
+ * [#997](https://github.com/pmd/pmd/issues/997): \[java] Java8 parsing corner case with annotated array types
+ * [#998](https://github.com/pmd/pmd/issues/998): \[java] AST inconsistencies around FormalParameter
+ * [#1019](https://github.com/pmd/pmd/issues/1019): \[java] Breaking Java Grammar changes for PMD 7.0.0
+ * [#1124](https://github.com/pmd/pmd/issues/1124): \[java] ImmutableList implementation in the qname codebase
+ * [#1128](https://github.com/pmd/pmd/issues/1128): \[java] Improve ASTLocalVariableDeclaration
+ * [#1150](https://github.com/pmd/pmd/issues/1150): \[java] ClassOrInterfaceType AST improvements
+ * [#1207](https://github.com/pmd/pmd/issues/1207): \[java] Resolve explicit types using FQCNs, without hitting the classloader
+ * [#1367](https://github.com/pmd/pmd/issues/1367): \[java] Parsing error on annotated inner class
+ * [#1661](https://github.com/pmd/pmd/issues/1661): \[java] About operator nodes
+ * [#2366](https://github.com/pmd/pmd/pull/2366): \[java] Remove qualified names
+ * [#2819](https://github.com/pmd/pmd/issues/2819): \[java] GLB bugs in pmd 7
+ * [#3642](https://github.com/pmd/pmd/issues/3642): \[java] Parse error on rare extra dimensions on method return type on annotation methods
+ * [#3763](https://github.com/pmd/pmd/issues/3763): \[java] Ambiguous reference error in valid code
+ * [#3749](https://github.com/pmd/pmd/issues/3749): \[java] Improve `isOverridden` in ASTMethodDeclaration
+ * [#3750](https://github.com/pmd/pmd/issues/3750): \[java] Make symbol table support instanceof pattern bindings
+ * [#3752](https://github.com/pmd/pmd/issues/3752): \[java] Expose annotations in symbol API
+ * [#4237](https://github.com/pmd/pmd/pull/4237): \[java] Cleanup handling of Java comments
+ * [#4317](https://github.com/pmd/pmd/issues/4317): \[java] Some AST nodes should not be TypeNodes
+ * [#4359](https://github.com/pmd/pmd/issues/4359): \[java] Type resolution fails with NPE when the scope is not a type declaration
+ * [#4367](https://github.com/pmd/pmd/issues/4367): \[java] Move testrule TypeResTest into internal
+* java-bestpractices
+ * [#342](https://github.com/pmd/pmd/issues/342): \[java] AccessorMethodGeneration: Name clash with another public field not properly handled
+ * [#755](https://github.com/pmd/pmd/issues/755): \[java] AccessorClassGeneration false positive for private constructors
+ * [#770](https://github.com/pmd/pmd/issues/770): \[java] UnusedPrivateMethod yields false positive for counter-variant arguments
+ * [#807](https://github.com/pmd/pmd/issues/807): \[java] AccessorMethodGeneration false positive with overloads
+ * [#833](https://github.com/pmd/pmd/issues/833): \[java] ForLoopCanBeForeach should consider iterating on this
+ * [#1189](https://github.com/pmd/pmd/issues/1189): \[java] UnusedPrivateMethod false positive from inner class via external class
+ * [#1205](https://github.com/pmd/pmd/issues/1205): \[java] Improve ConstantsInInterface message to mention alternatives
+ * [#1212](https://github.com/pmd/pmd/issues/1212): \[java] Don't raise JUnitTestContainsTooManyAsserts on JUnit 5's assertAll
+ * [#1422](https://github.com/pmd/pmd/issues/1422): \[java] JUnitTestsShouldIncludeAssert false positive with inherited @Rule field
+ * [#1455](https://github.com/pmd/pmd/issues/1455): \[java] JUnitTestsShouldIncludeAssert: False positives for assert methods named "check" and "verify"
+ * [#1563](https://github.com/pmd/pmd/issues/1563): \[java] ForLoopCanBeForeach false positive with method call using index variable
+ * [#1565](https://github.com/pmd/pmd/issues/1565): \[java] JUnitAssertionsShouldIncludeMessage false positive with AssertJ
+ * [#1747](https://github.com/pmd/pmd/issues/1747): \[java] PreserveStackTrace false-positive
+ * [#1969](https://github.com/pmd/pmd/issues/1969): \[java] MissingOverride false-positive triggered by package-private method overwritten in another package by extending class
+ * [#1998](https://github.com/pmd/pmd/issues/1998): \[java] AccessorClassGeneration false-negative: subclass calls private constructor
+ * [#2130](https://github.com/pmd/pmd/issues/2130): \[java] UnusedLocalVariable: false-negative with array
+ * [#2147](https://github.com/pmd/pmd/issues/2147): \[java] JUnitTestsShouldIncludeAssert - false positives with lambdas and static methods
+ * [#2464](https://github.com/pmd/pmd/issues/2464): \[java] LooseCoupling must ignore class literals: ArrayList.class
+ * [#2542](https://github.com/pmd/pmd/issues/2542): \[java] UseCollectionIsEmpty can not detect the case `foo.bar().size()`
+ * [#2650](https://github.com/pmd/pmd/issues/2650): \[java] UseTryWithResources false positive when AutoCloseable helper used
+ * [#2796](https://github.com/pmd/pmd/issues/2796): \[java] UnusedAssignment false positive with call chains
+ * [#2797](https://github.com/pmd/pmd/issues/2797): \[java] MissingOverride long-standing issues
+ * [#2806](https://github.com/pmd/pmd/issues/2806): \[java] SwitchStmtsShouldHaveDefault false-positive with Java 14 switch non-fallthrough branches
+ * [#2822](https://github.com/pmd/pmd/issues/2822): \[java] LooseCoupling rule: Extend to cover user defined implementations and interfaces
+ * [#2843](https://github.com/pmd/pmd/pull/2843): \[java] Fix UnusedAssignment FP with field accesses
+ * [#2882](https://github.com/pmd/pmd/issues/2882): \[java] UseTryWithResources - false negative for explicit close
+ * [#2883](https://github.com/pmd/pmd/issues/2883): \[java] JUnitAssertionsShouldIncludeMessage false positive with method call
+ * [#2890](https://github.com/pmd/pmd/issues/2890): \[java] UnusedPrivateMethod false positive with generics
+ * [#2946](https://github.com/pmd/pmd/issues/2946): \[java] SwitchStmtsShouldHaveDefault false positive on enum inside enums
+ * [#3672](https://github.com/pmd/pmd/pull/3672): \[java] LooseCoupling - fix false positive with generics
+ * [#3675](https://github.com/pmd/pmd/pull/3675): \[java] MissingOverride - fix false positive with mixing type vars
+ * [#3858](https://github.com/pmd/pmd/issues/3858): \[java] UseCollectionIsEmpty should infer local variable type from method invocation
+ * [#4516](https://github.com/pmd/pmd/issues/4516): \[java] UnusedLocalVariable: false-negative with try-with-resources
+ * [#4517](https://github.com/pmd/pmd/issues/4517): \[java] UnusedLocalVariable: false-negative with compound assignments
+ * [#4518](https://github.com/pmd/pmd/issues/4518): \[java] UnusedLocalVariable: false-positive with multiple for-loop indices
+* java-codestyle
+ * [#1208](https://github.com/pmd/pmd/issues/1208): \[java] PrematureDeclaration rule false-positive on variable declared to measure time
+ * [#1429](https://github.com/pmd/pmd/issues/1429): \[java] PrematureDeclaration as result of method call (false positive)
+ * [#1480](https://github.com/pmd/pmd/issues/1480): \[java] IdenticalCatchBranches false positive with return expressions
+ * [#1673](https://github.com/pmd/pmd/issues/1673): \[java] UselessParentheses false positive with conditional operator
+ * [#1790](https://github.com/pmd/pmd/issues/1790): \[java] UnnecessaryFullyQualifiedName false positive with enum constant
+ * [#1918](https://github.com/pmd/pmd/issues/1918): \[java] UselessParentheses false positive with boolean operators
+ * [#2134](https://github.com/pmd/pmd/issues/2134): \[java] PreserveStackTrace not handling `Throwable.addSuppressed(...)`
+ * [#2299](https://github.com/pmd/pmd/issues/2299): \[java] UnnecessaryFullyQualifiedName false positive with similar package name
+ * [#2391](https://github.com/pmd/pmd/issues/2391): \[java] UseDiamondOperator FP when expected type and constructed type have a different parameterization
+ * [#2528](https://github.com/pmd/pmd/issues/2528): \[java] MethodNamingConventions - JUnit 5 method naming not support ParameterizedTest
+ * [#2739](https://github.com/pmd/pmd/issues/2739): \[java] UselessParentheses false positive for string concatenation
+ * [#2748](https://github.com/pmd/pmd/issues/2748): \[java] UnnecessaryCast false positive with unchecked cast
+ * [#2973](https://github.com/pmd/pmd/issues/2973): \[java] New rule: UnnecessaryBoxing
+ * [#3195](https://github.com/pmd/pmd/pull/3195): \[java] Improve rule UnnecessaryReturn to detect more cases
+ * [#3218](https://github.com/pmd/pmd/pull/3218): \[java] Generalize UnnecessaryCast to flag all unnecessary casts
+ * [#3221](https://github.com/pmd/pmd/issues/3221): \[java] PrematureDeclaration false positive for unused variables
+ * [#3238](https://github.com/pmd/pmd/issues/3238): \[java] Improve ExprContext, fix FNs of UnnecessaryCast
+ * [#3500](https://github.com/pmd/pmd/pull/3500): \[java] UnnecessaryBoxing - check for Integer.valueOf(String) calls
+ * [#4273](https://github.com/pmd/pmd/issues/4273): \[java] CommentDefaultAccessModifier ignoredAnnotations should include "org.junit.jupiter.api.extension.RegisterExtension" by default
+ * [#4357](https://github.com/pmd/pmd/pull/4357): \[java] Fix IllegalStateException in UseDiamondOperator rule
+ * [#4487](https://github.com/pmd/pmd/issues/4487): \[java] UnnecessaryConstructor: false-positive with @Inject and @Autowired
+ * [#4511](https://github.com/pmd/pmd/issues/4511): \[java] LocalVariableCouldBeFinal shouldn't report unused variables
+ * [#4512](https://github.com/pmd/pmd/issues/4512): \[java] MethodArgumentCouldBeFinal shouldn't report unused parameters
+* java-design
+ * [#1014](https://github.com/pmd/pmd/issues/1014): \[java] LawOfDemeter: False positive with lambda expression
+ * [#1605](https://github.com/pmd/pmd/issues/1605): \[java] LawOfDemeter: False positive for standard UTF-8 charset name
+ * [#2160](https://github.com/pmd/pmd/issues/2160): \[java] Issues with Law of Demeter
+ * [#2175](https://github.com/pmd/pmd/issues/2175): \[java] LawOfDemeter: False positive for chained methods with generic method call
+ * [#2179](https://github.com/pmd/pmd/issues/2179): \[java] LawOfDemeter: False positive with static property access - should treat class-level property as global object, not dot-accessed property
+ * [#2180](https://github.com/pmd/pmd/issues/2180): \[java] LawOfDemeter: False positive with Thread and ThreadLocalRandom
+ * [#2182](https://github.com/pmd/pmd/issues/2182): \[java] LawOfDemeter: False positive with package-private access
+ * [#2188](https://github.com/pmd/pmd/issues/2188): \[java] LawOfDemeter: False positive with fields assigned to local vars
+ * [#2536](https://github.com/pmd/pmd/issues/2536): \[java] ClassWithOnlyPrivateConstructorsShouldBeFinal can't detect inner class
+ * [#3668](https://github.com/pmd/pmd/pull/3668): \[java] ClassWithOnlyPrivateConstructorsShouldBeFinal - fix FP with inner private classes
+ * [#3754](https://github.com/pmd/pmd/issues/3754): \[java] SingularField false positive with read in while condition
+ * [#3786](https://github.com/pmd/pmd/issues/3786): \[java] SimplifyBooleanReturns should consider operator precedence
+ * [#4238](https://github.com/pmd/pmd/pull/4238): \[java] Make LawOfDemeter not use the rulechain
+ * [#4254](https://github.com/pmd/pmd/issues/4254): \[java] ImmutableField - false positive with Lombok @Setter
+ * [#4477](https://github.com/pmd/pmd/issues/4477): \[java] SignatureDeclareThrowsException: false-positive with TestNG annotations
+ * [#4490](https://github.com/pmd/pmd/issues/4490): \[java] ImmutableField - false negative with Lombok @Getter
+* java-documentation
+ * [#4369](https://github.com/pmd/pmd/pull/4369): \[java] Improve CommentSize
+ * [#4416](https://github.com/pmd/pmd/pull/4416): \[java] Fix reported line number in CommentContentRule
+* java-errorprone
+ * [#659](https://github.com/pmd/pmd/issues/659): \[java] MissingBreakInSwitch - last default case does not contain a break
+ * [#1005](https://github.com/pmd/pmd/issues/1005): \[java] CloneMethodMustImplementCloneable triggers for interfaces
+ * [#1669](https://github.com/pmd/pmd/issues/1669): \[java] NullAssignment - FP with ternay and null as constructor argument
+ * [#1899](https://github.com/pmd/pmd/issues/1899): \[java] Recognize @SuppressWanings("fallthrough") for MissingBreakInSwitch
+ * [#2320](https://github.com/pmd/pmd/issues/2320): \[java] NullAssignment - FP with ternary and null as method argument
+ * [#2532](https://github.com/pmd/pmd/issues/2532): \[java] AvoidDecimalLiteralsInBigDecimalConstructor can not detect the case `new BigDecimal(Expression)`
+ * [#2579](https://github.com/pmd/pmd/issues/2579): \[java] MissingBreakInSwitch detects the lack of break in the last case
+ * [#2880](https://github.com/pmd/pmd/issues/2880): \[java] CompareObjectsWithEquals - false negative with type res
+ * [#2893](https://github.com/pmd/pmd/issues/2893): \[java] Remove special cases from rule EmptyCatchBlock
+ * [#2894](https://github.com/pmd/pmd/issues/2894): \[java] Improve MissingBreakInSwitch
+ * [#3071](https://github.com/pmd/pmd/issues/3071): \[java] BrokenNullCheck FP with PMD 6.30.0
+ * [#3087](https://github.com/pmd/pmd/issues/3087): \[java] UnnecessaryBooleanAssertion overlaps with SimplifiableTestAssertion
+ * [#3100](https://github.com/pmd/pmd/issues/3100): \[java] UseCorrectExceptionLogging FP in 6.31.0
+ * [#3173](https://github.com/pmd/pmd/issues/3173): \[java] UseProperClassLoader false positive
+ * [#3351](https://github.com/pmd/pmd/issues/3351): \[java] ConstructorCallsOverridableMethod ignores abstract methods
+ * [#3400](https://github.com/pmd/pmd/issues/3400): \[java] AvoidUsingOctalValues FN with underscores
+ * [#3843](https://github.com/pmd/pmd/issues/3843): \[java] UseEqualsToCompareStrings should consider return type
+ * [#4356](https://github.com/pmd/pmd/pull/4356): \[java] Fix NPE in CloseResourceRule
+ * [#4449](https://github.com/pmd/pmd/issues/4449): \[java] AvoidAccessibilityAlteration: Possible false positive in AvoidAccessibilityAlteration rule when using Lambda expression
+ * [#4493](https://github.com/pmd/pmd/issues/4493): \[java] MissingStaticMethodInNonInstantiatableClass: false-positive about @Inject
+ * [#4505](https://github.com/pmd/pmd/issues/4505): \[java] ImplicitSwitchFallThrough NPE in PMD 7.0.0-rc1
+ * [#4513](https://github.com/pmd/pmd/issues/4513): \[java] UselessOperationOnImmutable various false negatives with String
+ * [#4514](https://github.com/pmd/pmd/issues/4514): \[java] AvoidLiteralsInIfCondition false positive and negative for String literals when ignoreExpressions=true
+* java-multithreading
+ * [#2537](https://github.com/pmd/pmd/issues/2537): \[java] DontCallThreadRun can't detect the case that call run() in `this.run()`
+ * [#2538](https://github.com/pmd/pmd/issues/2538): \[java] DontCallThreadRun can't detect the case that call run() in `foo.bar.run()`
+ * [#2577](https://github.com/pmd/pmd/issues/2577): \[java] UseNotifyAllInsteadOfNotify falsely detect a special case with argument: `foo.notify(bar)`
+ * [#4483](https://github.com/pmd/pmd/issues/4483): \[java] NonThreadSafeSingleton false positive with double-checked locking
+* java-performance
+ * [#1224](https://github.com/pmd/pmd/issues/1224): \[java] InefficientEmptyStringCheck false negative in anonymous class
+ * [#2587](https://github.com/pmd/pmd/issues/2587): \[java] AvoidArrayLoops could also check for list copy through iterated List.add()
+ * [#2712](https://github.com/pmd/pmd/issues/2712): \[java] SimplifyStartsWith false-positive with AssertJ
+ * [#3486](https://github.com/pmd/pmd/pull/3486): \[java] InsufficientStringBufferDeclaration: Fix NPE
+ * [#3848](https://github.com/pmd/pmd/issues/3848): \[java] StringInstantiation: false negative when using method result
+* kotlin
+ * [#419](https://github.com/pmd/pmd/issues/419): \[kotlin] Add support for Kotlin
+ * [#4389](https://github.com/pmd/pmd/pull/4389): \[kotlin] Update grammar to version 1.8
+* swift
+ * [#1877](https://github.com/pmd/pmd/pull/1877): \[swift] Feature/swift rules
+ * [#1882](https://github.com/pmd/pmd/pull/1882): \[swift] UnavailableFunction Swift rule
+* xml
+ * [#1800](https://github.com/pmd/pmd/pull/1800): \[xml] Unimplement org.w3c.dom.Node from the XmlNodeWrapper
+
+### ✨ External Contributions
+
+* [#1658](https://github.com/pmd/pmd/pull/1658): \[core] Node support for Antlr-based languages - [Matías Fraga](https://github.com/matifraga) (@matifraga)
+* [#1698](https://github.com/pmd/pmd/pull/1698): \[core] [swift] Antlr Base Parser adapter and Swift Implementation - [Lucas Soncini](https://github.com/lsoncini) (@lsoncini)
+* [#1774](https://github.com/pmd/pmd/pull/1774): \[core] Antlr visitor rules - [Lucas Soncini](https://github.com/lsoncini) (@lsoncini)
+* [#1877](https://github.com/pmd/pmd/pull/1877): \[swift] Feature/swift rules - [Matías Fraga](https://github.com/matifraga) (@matifraga)
+* [#1881](https://github.com/pmd/pmd/pull/1881): \[doc] Add ANTLR documentation - [Matías Fraga](https://github.com/matifraga) (@matifraga)
+* [#1882](https://github.com/pmd/pmd/pull/1882): \[swift] UnavailableFunction Swift rule - [Tomás de Lucca](https://github.com/tomidelucca) (@tomidelucca)
+* [#2830](https://github.com/pmd/pmd/pull/2830): \[apex] Apexlink POC - [Kevin Jones](https://github.com/nawforce) (@nawforce)
+* [#3866](https://github.com/pmd/pmd/pull/3866): \[core] Add CLI Progress Bar - [@JerritEic](https://github.com/JerritEic) (@JerritEic)
+* [#4402](https://github.com/pmd/pmd/pull/4402): \[javascript] CPD: add support for Typescript using antlr4 grammar - [Paul Guyot](https://github.com/pguyot) (@pguyot)
+* [#4403](https://github.com/pmd/pmd/pull/4403): \[julia] CPD: Add support for Julia code duplication - [Wener](https://github.com/wener-tiobe) (@wener-tiobe)
+* [#4412](https://github.com/pmd/pmd/pull/4412): \[doc] Added new error msg to ConstantsInInterface - [David Ljunggren](https://github.com/dague1) (@dague1)
+* [#4428](https://github.com/pmd/pmd/pull/4428): \[apex] ApexBadCrypto bug fix for #4427 - inline detection of hard coded values - [Steven Stearns](https://github.com/sfdcsteve) (@sfdcsteve)
+* [#4444](https://github.com/pmd/pmd/pull/4444): \[java] CommentDefaultAccessModifier - ignore org.junit.jupiter.api.extension.RegisterExtension by default - [Nirvik Patel](https://github.com/nirvikpatel) (@nirvikpatel)
+* [#4450](https://github.com/pmd/pmd/pull/4450): \[java] Fix #4449 AvoidAccessibilityAlteration: Correctly handle Lambda expressions in PrivilegedAction scenarios - [Seren](https://github.com/mohui1999) (@mohui1999)
+* [#4452](https://github.com/pmd/pmd/pull/4452): \[doc] Update PMD_APEX_ROOT_DIRECTORY documentation reference - [nwcm](https://github.com/nwcm) (@nwcm)
+* [#4474](https://github.com/pmd/pmd/pull/4474): \[java] ImmutableField: False positive with lombok (fixes #4254) - [Pim van der Loos](https://github.com/PimvanderLoos) (@PimvanderLoos)
+* [#4488](https://github.com/pmd/pmd/pull/4488): \[java] Fix #4477: A false-positive about SignatureDeclareThrowsException - [AnnaDev](https://github.com/LynnBroe) (@LynnBroe)
+* [#4494](https://github.com/pmd/pmd/pull/4494): \[java] Fix #4487: A false-positive about UnnecessaryConstructor and @Inject and @Autowired - [AnnaDev](https://github.com/LynnBroe) (@LynnBroe)
+* [#4495](https://github.com/pmd/pmd/pull/4495): \[java] Fix #4493: false-positive about MissingStaticMethodInNonInstantiatableClass and @Inject - [AnnaDev](https://github.com/LynnBroe) (@LynnBroe)
+* [#4520](https://github.com/pmd/pmd/pull/4520): \[doc] Fix typo: missing closing quotation mark after CPD-END - [João Dinis Ferreira](https://github.com/joaodinissf) (@joaodinissf)
+
+### 📈 Stats
+* 4557 commits
+* 572 closed tickets & PRs
+* Days since last release: 35
+
+
+
## 25-March-2023 - 7.0.0-rc1
We're excited to bring you the next major version of PMD!
diff --git a/docs/pages/release_notes_pmd7.md b/docs/pages/release_notes_pmd7.md
index 08f285d210..5579225cd2 100644
--- a/docs/pages/release_notes_pmd7.md
+++ b/docs/pages/release_notes_pmd7.md
@@ -488,6 +488,20 @@ We are shipping the following rules:
Contributors: [Jeroen Borgers](https://github.com/jborgers) (@jborgers),
[Peter Paul Bakker](https://github.com/stokpop) (@stokpop)
+#### New: CPD support for TypeScript
+
+Thanks to a contribution, CPD now supports the TypeScript language. It is shipped
+with the rest of the JavaScript support in the module `pmd-javascript`.
+
+Contributors: [Paul Guyot](https://github.com/pguyot) (@pguyot)
+
+#### New: CPD support for Julia
+
+Thanks to a contribution, CPD now supports the Julia language. It is shipped
+in the new module `pmd-julia`.
+
+Contributors: [Wener](https://github.com/wener-tiobe) (@wener-tiobe)
+
### Changed: JavaScript support
The JS specific parser options have been removed. The parser now always retains comments and uses version ES6.
@@ -530,33 +544,116 @@ Related issue: [[core] Explicitly name all language versions (#4120)](https://gi
### Changed Rules
-**Java**
+**General changes**
-* {% rule "java/codestyle/UnnecessaryFullyQualifiedName" %}: the rule has two new properties,
+* All statistical rules (like ExcessiveClassLength, ExcessiveParameterList) have been simplified and unified.
+ The properties `topscore` and `sigma` have been removed. The property `minimum` is still there, however the type is not
+ a decimal number anymore but has been changed to an integer. This affects rules in the languages Apex, Java, PLSQL
+ and Velocity Template Language (vm):
+ * Apex: {% rule apex/design/ExcessiveClassLength %}, {% rule apex/design/ExcessiveParameterList %},
+ {% rule apex/design/ExcessivePublicCount %}, {% rule apex/design/NcssConstructorCount %},
+ {% rule apex/design/NcssMethodCount %}, {% rule apex/design/NcssTypeCount %}
+ * Java: {% rule java/design/ExcessiveImports %}, {% rule java/design/ExcessiveParameterList %},
+ {% rule java/design/ExcessivePublicCount %}, {% rule java/design/SwitchDensity %}
+ * PLSQL: {% rule plsql/design/ExcessiveMethodLength %}, {% rule plsql/design/ExcessiveObjectLength %},
+ {% rule plsql/design/ExcessivePackageBodyLength %}, {% rule plsql/design/ExcessivePackageSpecificationLength %},
+ {% rule plsql/design/ExcessiveParameterList %}, {% rule plsql/design/ExcessiveTypeLength %},
+ {% rule plsql/design/NcssMethodCount %}, {% rule plsql/design/NcssObjectCount %},
+ {% rule plsql/design/NPathComplexity %}
+ * VM: {% rule vm/design/ExcessiveTemplateLength %}
+
+* The general property `violationSuppressXPath` which is available for all rules to
+ [suppress warnings](pmd_userdocs_suppressing_warnings.html) now uses XPath version 3.1 by default.
+ This version of the XPath language is mostly identical to XPath 2.0. In PMD 6, XPath 1.0 has been used.
+ If you upgrade from PMD 6, you need to verify your `violationSuppressXPath` properties.
+
+**Apex General changes**
+
+* The properties `cc_categories`, `cc_remediation_points_multiplier`, `cc_block_highlighting` have been removed
+ from all rules. These properties have been deprecated since PMD 6.13.0.
+ See [issue #1648](https://github.com/pmd/pmd/issues/1648) for more details.
+
+**Java General changes**
+
+* Violations reported on methods or classes previously reported the line range of the entire method
+ or class. With PMD 7.0.0, the reported location is now just the identifier of the method or class.
+ This affects various rules, e.g. {% rule java/design/CognitiveComplexity %}.
+
+ The report location is controlled by the overrides of the method {% jdoc core::lang.ast.Node#getReportLocation() %}
+ in different node types.
+
+ See [issue #4439](https://github.com/pmd/pmd/issues/4439) and [issue #730](https://github.com/pmd/pmd/issues/730)
+ for more details.
+
+**Java Best Practices**
+
+* {% rule java/bestpractices/ArrayIsStoredDirectly %}: Violations are now reported on the assignment and not
+ anymore on the formal parameter. The reported line numbers will probably move.
+* {% rule java/bestpractices/AvoidReassigningLoopVariables %}: This rule might not report anymore all
+ reassignments of the control variable in for-loops when the property `forReassign` is set to `skip`.
+ See [issue #4500](https://github.com/pmd/pmd/issues/4500) for more details.
+* {% rule java/bestpractices/LooseCoupling %}: The rule has a new property to allow some types to be coupled
+ to (`allowedTypes`).
+* {% rule java/bestpractices/UnusedLocalVariable %}: This rule has some important false-negatives fixed
+ and finds many more cases now. For details see issues [#2130](https://github.com/pmd/pmd/issues/2130),
+ [#4516](https://github.com/pmd/pmd/issues/4516), and [#4517](https://github.com/pmd/pmd/issues/4517).
+
+**Java Codestyle**
+
+* {% rule java/codestyle/MethodNamingConventions %}: The property `checkNativeMethods` has been removed. The
+ property was deprecated since PMD 6.3.0. Use the property `nativePattern` to control whether native methods
+ should be considered or not.
+* {% rule java/codestyle/ShortVariable %}: This rule now also reports short enum constant names.
+* {% rule java/codestyle/UseDiamondOperator %}: The property `java7Compatibility` has been removed. The rule now
+ handles Java 7 properly without a property.
+* {% rule java/codestyle/UnnecessaryFullyQualifiedName %}: The rule has two new properties,
to selectively disable reporting on static field and method qualifiers. The rule also has been improved
to be more precise.
-* {% rule "java/codestyle/UselessParentheses" %}: the rule has two new properties which control how strict
+* {% rule java/codestyle/UselessParentheses %}: The rule has two new properties which control how strict
the rule should be applied. With `ignoreClarifying` (default: true) parentheses that are strictly speaking
not necessary are allowed, if they separate expressions of different precedence.
The other property `ignoreBalancing` (default: true) is similar, in that it allows parentheses that help
reading and understanding the expressions.
-* {% rule "java/bestpractices/LooseCoupling" %}: the rule has a new property to allow some types to be coupled
- to (`allowedTypes`).
-* {% rule "java/errorprone/EmptyCatchBlock" %}: `CloneNotSupportedException` and `InterruptedException` are not
- special-cased anymore. Rename the exception parameter to `ignored` to ignore them.
-* {% rule "java/errorprone/DontImportSun" %}: `sun.misc.Signal` is not special-cased anymore.
-* {% rule "java/codestyle/UseDiamondOperator" %}: the property `java7Compatibility` is removed. The rule now
- handles Java 7 properly without a property.
-* {% rule "java/design/SingularField" %}: Properties `checkInnerClasses` and `disallowNotAssignment` are removed.
- The rule is now more precise and will check these cases properly.
-* {% rule "java/design/UseUtilityClass" %}: The property `ignoredAnnotations` has been removed.
-* {% rule "java/design/LawOfDemeter" %}: the rule has a new property `trustRadius`. This defines the maximum degree
- of trusted data. The default of 1 is the most restrictive.
-* {% rule "java/documentation/CommentContent" %}: The properties `caseSensitive` and `disallowedTerms` are removed. The
- new property `fobiddenRegex` can be used now to define the disallowed terms with a single regular
- expression.
-* {% rule "java/design/ImmutableField" %}: the property `ignoredAnnotations` has been removed. The property was
+
+**Java Design**
+
+* {% rule java/design/CyclomaticComplexity %}: The property `reportLevel` has been removed. The property was
+ deprecated since PMD 6.0.0. The report level can now be configured separated for classes and methods using
+ `classReportLevel` and `methodReportLevel` instead.
+* {% rule java/design/ImmutableField %}: The property `ignoredAnnotations` has been removed. The property was
deprecated since PMD 6.52.0.
+* {% rule java/design/LawOfDemeter %}: The rule has a new property `trustRadius`. This defines the maximum degree
+ of trusted data. The default of 1 is the most restrictive.
+* {% rule java/design/NPathComplexity %}: The property `minimum` has been removed. It was deprecated since PMD 6.0.0.
+ Use the property `reportLevel` instead.
+* {% rule java/design/SingularField %}: The properties `checkInnerClasses` and `disallowNotAssignment` have been removed.
+ The rule is now more precise and will check these cases properly.
+* {% rule java/design/UseUtilityClass %}: The property `ignoredAnnotations` has been removed.
+
+**Java Documentation**
+
+* {% rule java/documentation/CommentContent %}: The properties `caseSensitive` and `disallowedTerms` are removed. The
+ new property `forbiddenRegex` can be used now to define the disallowed terms with a single regular
+ expression.
+* {% rule java/documentation/CommentRequired %}:
+ * Overridden methods are now detected even without the `@Override`
+ annotation. This is relevant for the property `methodWithOverrideCommentRequirement`.
+ See also [pull request #3757](https://github.com/pmd/pmd/pull/3757).
+ * Elements in annotation types are now detected as well. This might lead to an increased number of violations
+ for missing public method comments.
+* {% rule java/documentation/CommentSize %}: When determining the line-length of a comment, the leading comment
+ prefix markers (e.g. `*` or `//`) are ignored and don't add up to the line-length.
+ See also [pull request #4369](https://github.com/pmd/pmd/pull/4369).
+
+**Java Error Prone**
+
+* {% rule java/errorprone/AvoidDuplicateLiterals %}: The property `exceptionfile` has been removed. The property was
+ deprecated since PMD 6.10.0. Use the property `exceptionList` instead.
+* {% rule java/errorprone/DontImportSun %}: `sun.misc.Signal` is not special-cased anymore.
+* {% rule java/errorprone/EmptyCatchBlock %}: `CloneNotSupportedException` and `InterruptedException` are not
+ special-cased anymore. Rename the exception parameter to `ignored` to ignore them.
+* {% rule java/errorprone/ImplicitSwitchFallThrough %}: Violations are now reported on the case statements
+ rather than on the switch statements. This is more accurate but might result in more violations now.
### Deprecated Rules
diff --git a/pmd-ant/pom.xml b/pmd-ant/pom.xml
index 7001eeddfc..26e41e525d 100644
--- a/pmd-ant/pom.xml
+++ b/pmd-ant/pom.xml
@@ -3,9 +3,7 @@
~ BSD-style license; for more info see http://pmd.sourceforge.net/license.html
-->
-true
then PMD
- * will log debug information.
- *
- * @return true
if debug logging is enabled, false
- * otherwise.
- */
- public boolean isDebug() {
- return debug;
- }
-
- /**
- * Set the debug indicator.
- *
- * @param debug
- * The debug indicator to set.
- * @see #isDebug()
- */
- public void setDebug(boolean debug) {
- this.debug = debug;
- }
}
diff --git a/pmd-core/src/main/java/net/sourceforge/pmd/PMD.java b/pmd-core/src/main/java/net/sourceforge/pmd/PMD.java
deleted file mode 100644
index 46d7ff111c..0000000000
--- a/pmd-core/src/main/java/net/sourceforge/pmd/PMD.java
+++ /dev/null
@@ -1,251 +0,0 @@
-/**
- * BSD-style license; for more info see http://pmd.sourceforge.net/license.html
- */
-
-package net.sourceforge.pmd;
-
-import java.io.IOException;
-import java.io.OutputStreamWriter;
-import java.io.Writer;
-import java.util.Map.Entry;
-import java.util.Objects;
-
-import org.apache.commons.lang3.exception.ExceptionUtils;
-import org.checkerframework.checker.nullness.qual.NonNull;
-import org.slf4j.Logger;
-import org.slf4j.LoggerFactory;
-import org.slf4j.event.Level;
-
-import net.sourceforge.pmd.benchmark.TextTimingReportRenderer;
-import net.sourceforge.pmd.benchmark.TimeTracker;
-import net.sourceforge.pmd.benchmark.TimingReport;
-import net.sourceforge.pmd.benchmark.TimingReportRenderer;
-import net.sourceforge.pmd.cli.PMDCommandLineInterface;
-import net.sourceforge.pmd.cli.PmdParametersParseResult;
-import net.sourceforge.pmd.internal.LogMessages;
-import net.sourceforge.pmd.internal.Slf4jSimpleConfiguration;
-import net.sourceforge.pmd.reporting.ReportStats;
-import net.sourceforge.pmd.util.log.MessageReporter;
-import net.sourceforge.pmd.util.log.internal.SimpleMessageReporter;
-
-/**
- * Entry point for PMD's CLI. Use {@link #runPmd(PMDConfiguration)}
- * or {@link #runPmd(String...)} to mimic a CLI run. This class is
- * not a supported programmatic API anymore, use {@link PmdAnalysis}
- * for fine control over the PMD integration and execution.
- *
- * Warning: This class is not intended to be instantiated or subclassed. It will
- * be made final in PMD7.
- *
- * @deprecated This class is to be removed in PMD 7 in favor of a unified PmdCli entry point. {@link PmdAnalysis} should be used for non-CLI use-cases.
- */
-@Deprecated
-public final class PMD {
-
- private static final String PMD_PACKAGE = "net.sourceforge.pmd";
- // not final, in order to re-initialize logging
- private static Logger log = LoggerFactory.getLogger(PMD_PACKAGE);
-
- private PMD() {
- }
-
- /**
- * Entry to invoke PMD as command line tool. Note that this will
- * invoke {@link System#exit(int)}.
- *
- * @param args command line arguments
- */
- public static void main(String[] args) {
- StatusCode exitCode = runPmd(args);
- PMDCommandLineInterface.setStatusCodeOrExit(exitCode.toInt());
- }
-
- /**
- * Parses the command line arguments and executes PMD. Returns the
- * status code without exiting the VM. Note that the arguments parsing
- * may itself fail and produce a {@link StatusCode#ERROR}. This will
- * print the error message to standard error.
- *
- * @param args command line arguments
- *
- * @return the status code. Note that {@link PMDConfiguration#isFailOnViolation()}
- * (flag {@code --failOnViolation}) may turn an {@link StatusCode#OK} into a {@link
- * StatusCode#VIOLATIONS_FOUND}.
- */
- public static StatusCode runPmd(String... args) {
- PmdParametersParseResult parseResult = PmdParametersParseResult.extractParameters(args);
-
- // todo these warnings/errors should be output on a PmdRenderer
- if (!parseResult.getDeprecatedOptionsUsed().isEmpty()) {
- Entry There are several aspects to the configuration of PMD.
+ * The aspects related to generic PMD behavior: The aspects related to Rules and Source files are: The aspects related to special PMD behavior are:Rulesets
*
- *
- *
*
- * Source files
+ *
*
- *
*
+ * System.getProperty("file.encoding")
.
- * {@link #getSourceEncoding()}Rendering
+ *
*
*
*
- * Language configuration
*
- *
+ *
+ * Miscellaneous
+ *
+ *
*/
public class PMDConfiguration extends AbstractConfiguration {
@@ -113,7 +110,7 @@ public class PMDConfiguration extends AbstractConfiguration {
private ClassLoader classLoader = getClass().getClassLoader();
private final LanguageVersionDiscoverer languageVersionDiscoverer;
private LanguageVersion forceLanguageVersion;
- private MessageReporter reporter = new SimpleMessageReporter(LoggerFactory.getLogger(PMD.class));
+ private MessageReporter reporter = new SimpleMessageReporter(LoggerFactory.getLogger(PmdAnalysis.class));
// Rule and source file options
private Listfile://
) the file will be read with each line representing
* an entry on the classpath.
You can specify multiple class paths separated by `:` on Unix-systems or `;` under Windows.
+ * See {@link File#pathSeparator}.
+ *
* @param classpath The prepended classpath.
*
* @throws IllegalArgumentException if the given classpath is invalid (e.g. does not exist)
@@ -738,60 +734,6 @@ public class PMDConfiguration extends AbstractConfiguration {
this.reportProperties = reportProperties;
}
- /**
- * Return the stress test indicator. If this value is true
then
- * PMD will randomize the order of file processing to attempt to shake out
- * bugs.
- *
- * @return true
if stress test is enbaled, false
- * otherwise.
- *
- * @deprecated For removal
- */
- @Deprecated
- public boolean isStressTest() {
- return stressTest;
- }
-
- /**
- * Set the stress test indicator.
- *
- * @param stressTest
- * The stree test indicator to set.
- * @see #isStressTest()
- * @deprecated For removal.
- */
- @Deprecated
- public void setStressTest(boolean stressTest) {
- this.stressTest = stressTest;
- }
-
- /**
- * Return the benchmark indicator. If this value is true
then
- * PMD will log benchmark information.
- *
- * @return true
if benchmark logging is enbaled,
- * false
otherwise.
- * @deprecated This behavior is down to CLI, not part of the core analysis.
- */
- @Deprecated
- public boolean isBenchmark() {
- return benchmark;
- }
-
- /**
- * Set the benchmark indicator.
- *
- * @param benchmark
- * The benchmark indicator to set.
- * @see #isBenchmark()
- * @deprecated This behavior is down to CLI, not part of the core analysis.
- */
- @Deprecated
- public void setBenchmark(boolean benchmark) {
- this.benchmark = benchmark;
- }
-
/**
* Whether PMD should exit with status 4 (the default behavior, true) if
* violations are found or just with 0 (to not break the build, e.g.).
diff --git a/pmd-core/src/main/java/net/sourceforge/pmd/PmdAnalysis.java b/pmd-core/src/main/java/net/sourceforge/pmd/PmdAnalysis.java
index 1bf5e9a584..638d6b97de 100644
--- a/pmd-core/src/main/java/net/sourceforge/pmd/PmdAnalysis.java
+++ b/pmd-core/src/main/java/net/sourceforge/pmd/PmdAnalysis.java
@@ -44,6 +44,7 @@ import net.sourceforge.pmd.lang.document.FileCollector;
import net.sourceforge.pmd.lang.document.TextFile;
import net.sourceforge.pmd.renderers.Renderer;
import net.sourceforge.pmd.reporting.ConfigurableFileNameRenderer;
+import net.sourceforge.pmd.reporting.FileAnalysisListener;
import net.sourceforge.pmd.reporting.GlobalAnalysisListener;
import net.sourceforge.pmd.reporting.ListenerInitializer;
import net.sourceforge.pmd.reporting.ReportStats;
@@ -53,11 +54,19 @@ import net.sourceforge.pmd.util.StringUtil;
import net.sourceforge.pmd.util.log.MessageReporter;
/**
- * Main programmatic API of PMD. Create and configure a {@link PMDConfiguration},
+ * Main programmatic API of PMD. This is not a CLI entry point, see module
+ * {@code pmd-cli} for that.
+ *
+ *
{@code * PMDConfiguration config = new PMDConfiguration(); * config.setDefaultLanguageVersion(LanguageRegistry.findLanguageByTerseName("java").getVersion("11")); @@ -81,6 +90,42 @@ import net.sourceforge.pmd.util.log.MessageReporter; * } * }* + *
If you want strongly typed access to violations and other analysis events, + * you can implement and register a {@link GlobalAnalysisListener} with {@link #addListener(GlobalAnalysisListener)}. + * The listener needs to provide a new {@link FileAnalysisListener} for each file, + * which will receive events from the analysis. The listener's lifecycle + * happens only once the analysis is started ({@link #performAnalysis()}). + * + *
If you want access to all events once the analysis ends instead of processing + * events as they go, you can obtain a {@link Report} instance from {@link #performAnalysisAndCollectReport()}, + * or use {@link Report.GlobalReportBuilderListener} manually. Keep in + * mind collecting a report is less memory-efficient than using a listener. + * + *
If you want to process events in batches, one per file, you can + * use {@link Report.ReportBuilderListener}. to implement {@link GlobalAnalysisListener#startFileAnalysis(TextFile)}. + * + *
Listeners can be used alongside renderers. + * + *
The analysis reports messages like meta warnings and errors through a
+ * {@link MessageReporter} instance. To override how those messages are output,
+ * you can set it in {@link PMDConfiguration#setReporter(MessageReporter)}.
+ * By default, it forwards messages to SLF4J.
+ *
*/
public final class PmdAnalysis implements AutoCloseable {
diff --git a/pmd-core/src/main/java/net/sourceforge/pmd/cli/PMDCommandLineInterface.java b/pmd-core/src/main/java/net/sourceforge/pmd/cli/PMDCommandLineInterface.java
deleted file mode 100644
index 02f80e9cc7..0000000000
--- a/pmd-core/src/main/java/net/sourceforge/pmd/cli/PMDCommandLineInterface.java
+++ /dev/null
@@ -1,228 +0,0 @@
-/**
- * BSD-style license; for more info see http://pmd.sourceforge.net/license.html
- */
-
-package net.sourceforge.pmd.cli;
-
-import java.util.Properties;
-
-import net.sourceforge.pmd.PMD;
-import net.sourceforge.pmd.PMD.StatusCode;
-import net.sourceforge.pmd.PMDVersion;
-import net.sourceforge.pmd.annotation.InternalApi;
-import net.sourceforge.pmd.lang.Language;
-import net.sourceforge.pmd.lang.LanguageRegistry;
-import net.sourceforge.pmd.properties.PropertyDescriptor;
-import net.sourceforge.pmd.renderers.Renderer;
-import net.sourceforge.pmd.renderers.RendererFactory;
-
-import com.beust.jcommander.JCommander;
-import com.beust.jcommander.ParameterException;
-
-/**
- * @author Romain Pelisse <belaran@gmail.com>
- * @deprecated Internal API. Use {@link PMD#runPmd(String...)} or {@link PMD#main(String[])},
- * or {@link PmdParametersParseResult} if you just want to produce a configuration.
- */
-@Deprecated
-@InternalApi
-public final class PMDCommandLineInterface {
-
- @Deprecated
- public static final String PROG_NAME = "pmd";
-
- /**
- * @deprecated This is used for testing, but support for it will be removed in PMD 7.
- * Use {@link PMD#runPmd(String...)} or an overload to avoid exiting the VM. In PMD 7,
- * {@link PMD#main(String[])} will call {@link System#exit(int)} always.
- */
- @Deprecated
- public static final String NO_EXIT_AFTER_RUN = "net.sourceforge.pmd.cli.noExit";
-
- /**
- * @deprecated This is used for testing, but support for it will be removed in PMD 7.
- * Use {@link PMD#runPmd(String...)} or an overload to avoid exiting the VM. In PMD 7,
- * {@link PMD#main(String[])} will call {@link System#exit(int)} always.
- */
- @Deprecated
- public static final String STATUS_CODE_PROPERTY = "net.sourceforge.pmd.cli.status";
-
- /**
- * @deprecated Use {@link StatusCode#OK}
- */
- @Deprecated
- public static final int NO_ERRORS_STATUS = 0;
- /**
- * @deprecated Use {@link StatusCode#ERROR}
- */
- @Deprecated
- public static final int ERROR_STATUS = 1;
- /**
- * @deprecated Use {@link StatusCode#VIOLATIONS_FOUND}
- */
- @Deprecated
- public static final int VIOLATIONS_FOUND = 4;
-
- private PMDCommandLineInterface() { }
-
- /**
- * Note: this may terminate the VM.
- *
- * @deprecated Use {@link PmdParametersParseResult#extractParameters(String...)}
- */
- @Deprecated
- public static PMDParameters extractParameters(PMDParameters arguments, String[] args, String progName) {
- JCommander jcommander = new JCommander(arguments);
- jcommander.setProgramName(progName);
-
- try {
- jcommander.parse(args);
- if (arguments.isHelp()) {
- jcommander.usage();
- System.out.println(buildUsageText());
- setStatusCodeOrExit(NO_ERRORS_STATUS);
- }
- } catch (ParameterException e) {
- jcommander.usage();
- System.out.println(buildUsageText());
- System.err.println(e.getMessage());
- setStatusCodeOrExit(ERROR_STATUS);
- }
- return arguments;
- }
-
- public static String buildUsageText() {
- return "\n"
- + "Mandatory arguments:\n"
- + "1) A java source code filename or directory\n"
- + "2) A report format \n"
- + "3) A ruleset filename or a comma-delimited string of ruleset filenames\n"
- + "\n"
- + "For example: \n"
- + getWindowsLaunchCmd() + " -d c:\\my\\source\\code -f html -R java-unusedcode\n\n"
- + supportedVersions() + "\n"
- + "Available report formats and their configuration properties are:" + "\n" + getReports()
- + "\n" + getExamples() + "\n" + "\n" + "\n";
-
- }
-
- @Deprecated
- public static String buildUsageText(JCommander jcommander) {
- return buildUsageText();
- }
-
- private static String getExamples() {
- return getWindowsExample() + getUnixExample();
- }
-
- private static String getWindowsLaunchCmd() {
- final String WINDOWS_PROMPT = "C:\\>";
- final String launchCmd = "pmd-bin-" + PMDVersion.VERSION + "\\bin\\pmd.bat";
- return WINDOWS_PROMPT + launchCmd;
- }
-
- private static String getWindowsExample() {
- final String launchCmd = getWindowsLaunchCmd();
- final String WINDOWS_PATH_TO_CODE = "c:\\my\\source\\code ";
-
- return "For example on windows: " + "\n"
- + launchCmd + " --dir " + WINDOWS_PATH_TO_CODE
- + "--format text -R rulesets/java/quickstart.xml --use-version java-1.5 --debug" + "\n"
- + launchCmd + " -dir " + WINDOWS_PATH_TO_CODE
- + "-f xml --rulesets rulesets/java/quickstart.xml,category/java/codestyle.xml --encoding UTF-8" + "\n"
- + launchCmd + " --d " + WINDOWS_PATH_TO_CODE
- + "--rulesets rulesets/java/quickstart.xml --aux-classpath lib\\commons-collections.jar;lib\\derby.jar"
- + "\n"
- + launchCmd + " -d " + WINDOWS_PATH_TO_CODE
- + "-f html -R rulesets/java/quickstart.xml --aux-classpath file:///C:/my/classpathfile" + "\n" + "\n";
- }
-
- private static String getUnixExample() {
- final String launchCmd = "$ pmd-bin-" + PMDVersion.VERSION + "/bin/run.sh pmd";
- return "For example on *nix: " + "\n"
- + launchCmd
- + " --dir /home/workspace/src/main/java/code -f html --rulesets rulesets/java/quickstart.xml,category/java/codestyle.xml"
- + "\n"
- + launchCmd
- + " -d ./src/main/java/code -R rulesets/java/quickstart.xml -f xslt --property xsltFilename=my-own.xsl"
- + "\n"
- + launchCmd
- + " -d ./src/main/java/code -R rulesets/java/quickstart.xml -f xslt --property xsltFilename=html-report-v2.xslt"
- + "\n"
- + " - html-report-v2.xslt is at https://github.com/pmd/pmd/tree/master/pmd-core/etc/xslt/html-report-v2.xslt"
- + launchCmd
- + " -d ./src/main/java/code -f html -R rulesets/java/quickstart.xml --aux-classpath commons-collections.jar:derby.jar"
- + "\n";
- }
-
- private static String supportedVersions() {
- return "Languages and version supported:" + "\n"
- + LanguageRegistry.PMD.commaSeparatedList(Language::getId)
- + "\n";
- }
-
- /**
- * For testing purpose only...
- *
- * @param args
- *
- * @deprecated Use {@link PMD#runPmd(String...)}
- */
- @Deprecated
- public static void main(String[] args) {
- System.out.println(PMDCommandLineInterface.buildUsageText());
- }
-
- public static String jarName() {
- return "pmd-" + PMDVersion.VERSION + ".jar";
- }
-
- private static String getReports() {
- StringBuilder buf = new StringBuilder();
- for (String reportName : RendererFactory.supportedRenderers()) {
- Renderer renderer = RendererFactory.createRenderer(reportName, new Properties());
- buf.append(" ").append(reportName).append(": ");
- if (!reportName.equals(renderer.getName())) {
- buf.append(" Deprecated alias for '").append(renderer.getName()).append("\n");
- continue;
- }
- buf.append(renderer.getDescription()).append("\n");
-
- for (PropertyDescriptor> property : renderer.getPropertyDescriptors()) {
- buf.append(" ").append(property.name()).append(" - ");
- buf.append(property.description());
- Object deflt = property.defaultValue();
- if (deflt != null) {
- buf.append(" default: ").append(deflt);
- }
- buf.append("\n");
- }
-
- }
- return buf.toString();
- }
-
- public static void setStatusCodeOrExit(int status) {
- if (isExitAfterRunSet()) {
- System.exit(status);
- } else {
- setStatusCode(status);
- }
- }
-
- private static boolean isExitAfterRunSet() {
- String noExit = System.getenv(NO_EXIT_AFTER_RUN);
- if (noExit == null) {
- noExit = System.getProperty(NO_EXIT_AFTER_RUN);
- }
- return noExit == null;
- }
-
- private static void setStatusCode(int statusCode) {
- System.setProperty(STATUS_CODE_PROPERTY, Integer.toString(statusCode));
- }
-
- public static void printJcommanderUsageOnConsole() {
- new JCommander(new PMDParameters()).usage();
- }
-}
diff --git a/pmd-core/src/main/java/net/sourceforge/pmd/cli/PMDParameters.java b/pmd-core/src/main/java/net/sourceforge/pmd/cli/PMDParameters.java
deleted file mode 100644
index 2c96b29c8b..0000000000
--- a/pmd-core/src/main/java/net/sourceforge/pmd/cli/PMDParameters.java
+++ /dev/null
@@ -1,459 +0,0 @@
-/**
- * BSD-style license; for more info see http://pmd.sourceforge.net/license.html
- */
-
-package net.sourceforge.pmd.cli;
-
-import java.nio.file.Files;
-import java.nio.file.Path;
-import java.nio.file.Paths;
-import java.util.ArrayList;
-import java.util.Arrays;
-import java.util.List;
-import java.util.Properties;
-import java.util.stream.Collectors;
-
-import org.apache.commons.lang3.StringUtils;
-import org.checkerframework.checker.nullness.qual.NonNull;
-import org.checkerframework.checker.nullness.qual.Nullable;
-
-import net.sourceforge.pmd.PMD;
-import net.sourceforge.pmd.PMDConfiguration;
-import net.sourceforge.pmd.RulePriority;
-import net.sourceforge.pmd.annotation.InternalApi;
-import net.sourceforge.pmd.lang.Language;
-import net.sourceforge.pmd.lang.LanguageRegistry;
-import net.sourceforge.pmd.lang.LanguageVersion;
-
-import com.beust.jcommander.IStringConverter;
-import com.beust.jcommander.IValueValidator;
-import com.beust.jcommander.Parameter;
-import com.beust.jcommander.ParameterException;
-import com.beust.jcommander.validators.PositiveInteger;
-
-/**
- * @deprecated Internal API. Use {@link PMD#runPmd(String[])} or {@link PMD#main(String[])}
- */
-@Deprecated
-@InternalApi
-public class PMDParameters {
-
- static final String RELATIVIZE_PATHS_WITH = "--relativize-paths-with";
- @Parameter(names = { "--rulesets", "-rulesets", "-R" },
- description = "Path to a ruleset xml file. "
- + "The path may reference a resource on the classpath of the application, be a local file system path, or a URL. "
- + "The option can be repeated, and multiple arguments can be provided to a single occurrence of the option.",
- required = true,
- variableArity = true)
- private List A renderer does not have to use this method to output paths.
+ * Some report formats require a specific format for paths, eg URIs.
+ * They can implement this ad-hoc.
*/
- protected String determineFileName(FileId fileId) {
+ protected final String determineFileName(FileId fileId) {
return fileNameRenderer.getDisplayName(fileId);
}
diff --git a/pmd-core/src/main/java/net/sourceforge/pmd/renderers/Renderer.java b/pmd-core/src/main/java/net/sourceforge/pmd/renderers/Renderer.java
index ff84c4eff5..443eef1b1d 100644
--- a/pmd-core/src/main/java/net/sourceforge/pmd/renderers/Renderer.java
+++ b/pmd-core/src/main/java/net/sourceforge/pmd/renderers/Renderer.java
@@ -110,13 +110,20 @@ public interface Renderer extends PropertySource {
*/
Writer getWriter();
+ /**
+ * Set the {@link FileNameRenderer} used to render file paths to the report.
+ * Note that this renderer does not have to use the parameter to output paths.
+ * Some report formats require a specific format for paths (eg a URI), and are
+ * allowed to circumvent the provided strategy.
+ *
+ * @param fileNameRenderer a non-null file name renderer
+ */
void setFileNameRenderer(FileNameRenderer fileNameRenderer);
/**
* Set the Writer for the Renderer.
*
- * @param writer
- * The Writer.
+ * @param writer The Writer.
*/
void setWriter(Writer writer);
diff --git a/pmd-core/src/main/java/net/sourceforge/pmd/reporting/FileAnalysisListener.java b/pmd-core/src/main/java/net/sourceforge/pmd/reporting/FileAnalysisListener.java
index f882ac7632..7ecc79018e 100644
--- a/pmd-core/src/main/java/net/sourceforge/pmd/reporting/FileAnalysisListener.java
+++ b/pmd-core/src/main/java/net/sourceforge/pmd/reporting/FileAnalysisListener.java
@@ -8,6 +8,7 @@ import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
+import net.sourceforge.pmd.Report;
import net.sourceforge.pmd.Report.ProcessingError;
import net.sourceforge.pmd.Report.SuppressedViolation;
import net.sourceforge.pmd.RuleViolation;
@@ -17,10 +18,13 @@ import net.sourceforge.pmd.util.AssertionUtil;
/**
* A handler for events occuring during analysis of a single file. Instances
* are only used on a single thread for their entire lifetime, so don't
- * need to be synchronized to access state they own.
+ * need to be synchronized to access state they own. File listeners are
+ * spawned by a {@link GlobalAnalysisListener}.
*
* Listeners are assumed to be ready to receive events as soon as they
* are constructed.
+ *
+ * @see Report.ReportBuilderListener
*/
public interface FileAnalysisListener extends AutoCloseable {
diff --git a/pmd-core/src/test/java/net/sourceforge/pmd/PmdConfigurationTest.java b/pmd-core/src/test/java/net/sourceforge/pmd/PmdConfigurationTest.java
index 7fdc4933f6..210bef744c 100644
--- a/pmd-core/src/test/java/net/sourceforge/pmd/PmdConfigurationTest.java
+++ b/pmd-core/src/test/java/net/sourceforge/pmd/PmdConfigurationTest.java
@@ -216,30 +216,6 @@ class PmdConfigurationTest {
assertEquals(0, configuration.getReportProperties().size(), "Replaced report properties size");
}
- @Test
- void testDebug() {
- PMDConfiguration configuration = new PMDConfiguration();
- assertEquals(false, configuration.isDebug(), "Default debug");
- configuration.setDebug(true);
- assertEquals(true, configuration.isDebug(), "Changed debug");
- }
-
- @Test
- void testStressTest() {
- PMDConfiguration configuration = new PMDConfiguration();
- assertEquals(false, configuration.isStressTest(), "Default stress test");
- configuration.setStressTest(true);
- assertEquals(true, configuration.isStressTest(), "Changed stress test");
- }
-
- @Test
- void testBenchmark() {
- PMDConfiguration configuration = new PMDConfiguration();
- assertEquals(false, configuration.isBenchmark(), "Default benchmark");
- configuration.setBenchmark(true);
- assertEquals(true, configuration.isBenchmark(), "Changed benchmark");
- }
-
@Test
void testAnalysisCache(@TempDir Path folder) throws IOException {
final PMDConfiguration configuration = new PMDConfiguration();
diff --git a/pmd-core/src/test/java/net/sourceforge/pmd/cli/PMDCommandLineInterfaceTest.java b/pmd-core/src/test/java/net/sourceforge/pmd/cli/PMDCommandLineInterfaceTest.java
deleted file mode 100644
index 4b3e03f256..0000000000
--- a/pmd-core/src/test/java/net/sourceforge/pmd/cli/PMDCommandLineInterfaceTest.java
+++ /dev/null
@@ -1,110 +0,0 @@
-/**
- * BSD-style license; for more info see http://pmd.sourceforge.net/license.html
- */
-
-package net.sourceforge.pmd.cli;
-
-import static org.hamcrest.MatcherAssert.assertThat;
-import static org.hamcrest.Matchers.empty;
-import static org.junit.jupiter.api.Assertions.assertEquals;
-import static org.junit.jupiter.api.Assertions.assertNotNull;
-import static org.junit.jupiter.api.Assertions.assertTrue;
-
-import org.junit.jupiter.api.BeforeEach;
-import org.junit.jupiter.api.Test;
-
-import net.sourceforge.pmd.PMDConfiguration;
-import net.sourceforge.pmd.cache.NoopAnalysisCache;
-import net.sourceforge.pmd.lang.LanguageRegistry;
-
-import com.github.stefanbirkner.systemlambda.SystemLambda;
-
-
-/**
- * Unit test for {@link PMDCommandLineInterface}
- */
-class PMDCommandLineInterfaceTest {
-
- @BeforeEach
- void clearSystemProperties() {
- System.clearProperty(PMDCommandLineInterface.NO_EXIT_AFTER_RUN);
- System.clearProperty(PMDCommandLineInterface.STATUS_CODE_PROPERTY);
- }
-
- @Test
- void testProperties() {
- PMDParameters params = new PMDParameters();
- String[] args = { "-d", "source_folder", "-f", "yahtml", "-P", "outputDir=output_folder", "-R", "java-empty", };
- PMDCommandLineInterface.extractParameters(params, args, "PMD");
-
- assertEquals("output_folder", params.getProperties().getProperty("outputDir"));
- }
-
- @Test
- void testMultipleProperties() {
- PMDParameters params = new PMDParameters();
- String[] args = { "-d", "source_folder", "-f", "ideaj", "-P", "sourcePath=/home/user/source/", "-P",
- "fileName=Foo.java", "-P", "classAndMethodName=Foo.method", "-R", "java-empty", };
- PMDCommandLineInterface.extractParameters(params, args, "PMD");
-
- assertEquals("/home/user/source/", params.getProperties().getProperty("sourcePath"));
- assertEquals("Foo.java", params.getProperties().getProperty("fileName"));
- assertEquals("Foo.method", params.getProperties().getProperty("classAndMethodName"));
- }
-
-
- @Test
- void testNoCacheSwitch() {
- PMDParameters params = new PMDParameters();
- String[] args = {"-d", "source_folder", "-f", "ideaj", "-R", "java-empty", "-cache", "/home/user/.pmd/cache", "-no-cache", };
- PMDCommandLineInterface.extractParameters(params, args, "PMD");
-
- assertTrue(params.isIgnoreIncrementalAnalysis());
- PMDConfiguration config = params.toConfiguration(LanguageRegistry.PMD);
- assertTrue(config.isIgnoreIncrementalAnalysis());
- assertTrue(config.getAnalysisCache() instanceof NoopAnalysisCache);
- }
-
- @Test
- void testNoCacheSwitchLongOption() {
- PMDParameters params = new PMDParameters();
- String[] args = {"-d", "source_folder", "-f", "ideaj", "-R", "java-empty", "--cache", "/home/user/.pmd/cache", "--no-cache", };
- PMDCommandLineInterface.extractParameters(params, args, "PMD");
-
- assertTrue(params.isIgnoreIncrementalAnalysis());
- PMDConfiguration config = params.toConfiguration();
- assertTrue(config.isIgnoreIncrementalAnalysis());
- assertTrue(config.getAnalysisCache() instanceof NoopAnalysisCache);
- }
-
- @Test
- void testSetStatusCodeOrExitDoExit() throws Exception {
- int code = SystemLambda.catchSystemExit(() -> PMDCommandLineInterface.setStatusCodeOrExit(0));
- assertEquals(0, code);
- }
-
- @Test
- void testSetStatusCodeOrExitSetStatus() {
- System.setProperty(PMDCommandLineInterface.NO_EXIT_AFTER_RUN, "1");
-
- PMDCommandLineInterface.setStatusCodeOrExit(0);
- assertEquals(System.getProperty(PMDCommandLineInterface.STATUS_CODE_PROPERTY), "0");
- }
-
- @Test
- void testBuildUsageText() {
- // no exception..
- assertNotNull(PMDCommandLineInterface.buildUsageText());
- }
-
- @Test
- void testOnlyFileListOption() {
- PMDParameters params = new PMDParameters();
- String[] args = {"--file-list", "pmd.filelist", "-f", "text", "-R", "rulesets/java/quickstart.xml", "--no-cache", };
- PMDCommandLineInterface.extractParameters(params, args, "PMD");
-
- PMDConfiguration config = params.toConfiguration();
- assertEquals("pmd.filelist", config.getInputFile().toString());
- assertThat(config.getInputPathList(), empty()); // no additional input paths
- }
-}
diff --git a/pmd-core/src/test/java/net/sourceforge/pmd/cli/PMDParametersTest.java b/pmd-core/src/test/java/net/sourceforge/pmd/cli/PMDParametersTest.java
deleted file mode 100644
index 67fdbaef77..0000000000
--- a/pmd-core/src/test/java/net/sourceforge/pmd/cli/PMDParametersTest.java
+++ /dev/null
@@ -1,76 +0,0 @@
-/**
- * BSD-style license; for more info see http://pmd.sourceforge.net/license.html
- */
-
-package net.sourceforge.pmd.cli;
-
-import static net.sourceforge.pmd.util.CollectionUtil.listOf;
-import static org.junit.jupiter.api.Assertions.assertEquals;
-import static org.junit.jupiter.api.Assertions.assertFalse;
-import static org.junit.jupiter.api.Assertions.assertTrue;
-
-import java.nio.file.Path;
-
-import org.junit.jupiter.api.Test;
-
-import net.sourceforge.pmd.PMDConfiguration;
-import net.sourceforge.pmd.util.CollectionUtil;
-
-class PMDParametersTest {
-
- @Test
- void testMultipleDirsAndRuleSets() {
- PmdParametersParseResult result = PmdParametersParseResult.extractParameters(
- "-d", "a", "b", "-R", "x.xml", "y.xml"
- );
- assertMultipleDirsAndRulesets(result);
- }
-
- @Test
- void testMultipleDirsAndRuleSetsWithCommas() {
- PmdParametersParseResult result = PmdParametersParseResult.extractParameters(
- "-d", "a,b", "-R", "x.xml,y.xml"
- );
- assertMultipleDirsAndRulesets(result);
- }
-
- @Test
- void testMultipleDirsAndRuleSetsWithRepeatedOption() {
- PmdParametersParseResult result = PmdParametersParseResult.extractParameters(
- "-d", "a", "-d", "b", "-R", "x.xml", "-R", "y.xml"
- );
- assertMultipleDirsAndRulesets(result);
- }
-
- @Test
- void testNoPositionalParametersAllowed() {
- assertError(
- // vvvv
- "-R", "x.xml", "-d", "a", "--", "-d", "b"
- );
- }
-
-
- private void assertMultipleDirsAndRulesets(PmdParametersParseResult result) {
- assertFalse(result.isError());
- PMDConfiguration config = result.toConfiguration();
- assertEquals(CollectionUtil.map(config.getInputPathList(), Path::toString), listOf("a", "b"));
- assertEquals(config.getRuleSetPaths(), listOf("x.xml", "y.xml"));
- }
-
- @Test
- void testEmptyDirOption() {
- assertError("-d", "-R", "y.xml");
- }
-
- @Test
- void testEmptyRulesetOption() {
- assertError("-R", "-d", "something");
- }
-
- private void assertError(String... params) {
- PmdParametersParseResult result = PmdParametersParseResult.extractParameters(params);
- assertTrue(result.isError());
- }
-
-}
diff --git a/pmd-core/src/test/java/net/sourceforge/pmd/lang/LanguageParameterTest.java b/pmd-core/src/test/java/net/sourceforge/pmd/lang/LanguageParameterTest.java
deleted file mode 100644
index 1033dc9242..0000000000
--- a/pmd-core/src/test/java/net/sourceforge/pmd/lang/LanguageParameterTest.java
+++ /dev/null
@@ -1,27 +0,0 @@
-/**
- * BSD-style license; for more info see http://pmd.sourceforge.net/license.html
- */
-
-package net.sourceforge.pmd.lang;
-
-import static org.junit.jupiter.api.Assertions.assertEquals;
-
-import org.junit.jupiter.api.Test;
-
-import net.sourceforge.pmd.cli.PMDCommandLineInterface;
-import net.sourceforge.pmd.cli.PMDParameters;
-
-class LanguageParameterTest {
-
- /** Test that language parameters from the CLI are correctly passed through to the PMDConfiguration. Although this is a
- * CLI test, it resides here to take advantage of {@link net.sourceforge.pmd.lang.DummyLanguageModule}
- */
- @Test
- void testLanguageFromCliToConfiguration() {
- PMDParameters params = new PMDParameters();
- String[] args = { "-d", "source_folder", "-f", "ideaj", "-P", "sourcePath=/home/user/source/", "-R", "java-empty", "-force-language", "dummy"};
- PMDCommandLineInterface.extractParameters(params, args, "PMD");
-
- assertEquals(DummyLanguageModule.getInstance().getDefaultVersion().getName(), params.toConfiguration().getForceLanguageVersion().getName());
- }
-}
diff --git a/pmd-cpp/pom.xml b/pmd-cpp/pom.xml
index beb01403db..4f64a2da65 100644
--- a/pmd-cpp/pom.xml
+++ b/pmd-cpp/pom.xml
@@ -34,7 +34,7 @@
> {
- @Override
- public void validate(String name, List
> listStack = new ArrayDeque<>(0);
+ // 1-element "stacks", push must be followed by pop
+ private @Nullable JTypeMirror type;
+ private @Nullable List
@@ -440,12 +469,12 @@ public class UsedLocalVar {
+
@@ -30,7 +31,7 @@ public class Foo {
+
+
+
+
+