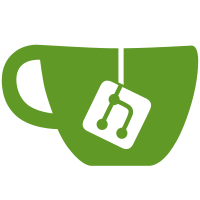
## Description The whole completion / snippets / AI is very tricky: - multiple snippet engines - native snippets on > 0.11 set their own keymaps, but not on 0.10 - multiple completion engines, like `nvim-cmp` and `blink.cmp` - multiple ai completion engines that have a different API - user's preference of showing ai suggestions as completion or not - none of the ai completion engines currently set undo points, which is bad Solution: - [x] added `LazyVim.cmp.actions`, where snippet engines and ai engines can register their action. - [x] an action returns `true` if it succeeded, or `false|nil` otherwise - [x] in a completion engine, we then try running multiple actions and use the fallback if needed - [x] so `<tab>` runs `{"snippet_forward", "ai_accept", "fallback"}` - [x] added `vim.g.ai_cmp`. When `true` we try to integrate the AI source in the completion engine. - [x] when `false`, `<tab>` should be used to insert the AI suggestion - [x] when `false`, the completion engine's ghost text is disabled - [x] luasnip support for blink (only works with blink `main`) - [x] create undo points when accepting AI suggestions ## Test Matrix | completion | snippets | ai | ai_cmp | tested? | |--------------|--------------|-------------|--------|---------| | nvim-cmp | native | copilot | true | ✅ | | nvim-cmp | native | copilot | false | ✅ | | nvim-cmp | native | codeium | true | ✅ | | nvim-cmp | native | codeium | false | ✅ | | nvim-cmp | luasnip | copilot | true | ✅ | | nvim-cmp | luasnip | copilot | false | ✅ | | nvim-cmp | luasnip | codeium | true | ✅ | | nvim-cmp | luasnip | codeium | false | ✅ | | blink.cmp | native | copilot | true | ✅ | | blink.cmp | native | copilot | false | ✅ | | blink.cmp | native | codeium | true | ✅ | | blink.cmp | native | codeium | false | ✅ | | blink.cmp | luasnip | copilot | true | ✅ | | blink.cmp | luasnip | copilot | false | ✅ | | blink.cmp | luasnip | codeium | true | ✅ | | blink.cmp | luasnip | codeium | false | ✅ | ## Related Issue(s) - [ ] Closes #4702 ## Screenshots <!-- Add screenshots of the changes if applicable. --> ## Checklist - [ ] I've read the [CONTRIBUTING](https://github.com/LazyVim/LazyVim/blob/main/CONTRIBUTING.md) guidelines.
204 lines
6.4 KiB
Lua
204 lines
6.4 KiB
Lua
return {
|
|
|
|
-- auto completion
|
|
{
|
|
"hrsh7th/nvim-cmp",
|
|
version = false, -- last release is way too old
|
|
event = "InsertEnter",
|
|
dependencies = {
|
|
"hrsh7th/cmp-nvim-lsp",
|
|
"hrsh7th/cmp-buffer",
|
|
"hrsh7th/cmp-path",
|
|
},
|
|
-- Not all LSP servers add brackets when completing a function.
|
|
-- To better deal with this, LazyVim adds a custom option to cmp,
|
|
-- that you can configure. For example:
|
|
--
|
|
-- ```lua
|
|
-- opts = {
|
|
-- auto_brackets = { "python" }
|
|
-- }
|
|
-- ```
|
|
opts = function()
|
|
vim.api.nvim_set_hl(0, "CmpGhostText", { link = "Comment", default = true })
|
|
local cmp = require("cmp")
|
|
local defaults = require("cmp.config.default")()
|
|
local auto_select = true
|
|
return {
|
|
auto_brackets = {}, -- configure any filetype to auto add brackets
|
|
completion = {
|
|
completeopt = "menu,menuone,noinsert" .. (auto_select and "" or ",noselect"),
|
|
},
|
|
preselect = auto_select and cmp.PreselectMode.Item or cmp.PreselectMode.None,
|
|
mapping = cmp.mapping.preset.insert({
|
|
["<C-b>"] = cmp.mapping.scroll_docs(-4),
|
|
["<C-f>"] = cmp.mapping.scroll_docs(4),
|
|
["<C-n>"] = cmp.mapping.select_next_item({ behavior = cmp.SelectBehavior.Insert }),
|
|
["<C-p>"] = cmp.mapping.select_prev_item({ behavior = cmp.SelectBehavior.Insert }),
|
|
["<C-Space>"] = cmp.mapping.complete(),
|
|
["<CR>"] = LazyVim.cmp.confirm({ select = auto_select }),
|
|
["<C-y>"] = LazyVim.cmp.confirm({ select = true }),
|
|
["<S-CR>"] = LazyVim.cmp.confirm({ behavior = cmp.ConfirmBehavior.Replace }), -- Accept currently selected item. Set `select` to `false` to only confirm explicitly selected items.
|
|
["<C-CR>"] = function(fallback)
|
|
cmp.abort()
|
|
fallback()
|
|
end,
|
|
["<tab>"] = function(fallback)
|
|
return LazyVim.cmp.map({ "snippet_forward", "ai_accept" }, fallback)()
|
|
end,
|
|
}),
|
|
sources = cmp.config.sources({
|
|
{ name = "nvim_lsp" },
|
|
{ name = "path" },
|
|
}, {
|
|
{ name = "buffer" },
|
|
}),
|
|
formatting = {
|
|
format = function(entry, item)
|
|
local icons = LazyVim.config.icons.kinds
|
|
if icons[item.kind] then
|
|
item.kind = icons[item.kind] .. item.kind
|
|
end
|
|
|
|
local widths = {
|
|
abbr = vim.g.cmp_widths and vim.g.cmp_widths.abbr or 40,
|
|
menu = vim.g.cmp_widths and vim.g.cmp_widths.menu or 30,
|
|
}
|
|
|
|
for key, width in pairs(widths) do
|
|
if item[key] and vim.fn.strdisplaywidth(item[key]) > width then
|
|
item[key] = vim.fn.strcharpart(item[key], 0, width - 1) .. "…"
|
|
end
|
|
end
|
|
|
|
return item
|
|
end,
|
|
},
|
|
experimental = {
|
|
-- only show ghost text when we show ai completions
|
|
ghost_text = vim.g.ai_cmp and {
|
|
hl_group = "CmpGhostText",
|
|
} or false,
|
|
},
|
|
sorting = defaults.sorting,
|
|
}
|
|
end,
|
|
main = "lazyvim.util.cmp",
|
|
},
|
|
|
|
-- snippets
|
|
{
|
|
"nvim-cmp",
|
|
optional = true,
|
|
dependencies = {
|
|
{
|
|
"garymjr/nvim-snippets",
|
|
opts = {
|
|
friendly_snippets = true,
|
|
},
|
|
dependencies = { "rafamadriz/friendly-snippets" },
|
|
},
|
|
},
|
|
opts = function(_, opts)
|
|
opts.snippet = {
|
|
expand = function(item)
|
|
return LazyVim.cmp.expand(item.body)
|
|
end,
|
|
}
|
|
if LazyVim.has("nvim-snippets") then
|
|
table.insert(opts.sources, { name = "snippets" })
|
|
end
|
|
end,
|
|
},
|
|
|
|
-- auto pairs
|
|
{
|
|
"echasnovski/mini.pairs",
|
|
event = "VeryLazy",
|
|
opts = {
|
|
modes = { insert = true, command = true, terminal = false },
|
|
-- skip autopair when next character is one of these
|
|
skip_next = [=[[%w%%%'%[%"%.%`%$]]=],
|
|
-- skip autopair when the cursor is inside these treesitter nodes
|
|
skip_ts = { "string" },
|
|
-- skip autopair when next character is closing pair
|
|
-- and there are more closing pairs than opening pairs
|
|
skip_unbalanced = true,
|
|
-- better deal with markdown code blocks
|
|
markdown = true,
|
|
},
|
|
config = function(_, opts)
|
|
LazyVim.mini.pairs(opts)
|
|
end,
|
|
},
|
|
|
|
-- comments
|
|
{
|
|
"folke/ts-comments.nvim",
|
|
event = "VeryLazy",
|
|
opts = {},
|
|
},
|
|
|
|
-- Better text-objects
|
|
{
|
|
"echasnovski/mini.ai",
|
|
event = "VeryLazy",
|
|
opts = function()
|
|
local ai = require("mini.ai")
|
|
return {
|
|
n_lines = 500,
|
|
custom_textobjects = {
|
|
o = ai.gen_spec.treesitter({ -- code block
|
|
a = { "@block.outer", "@conditional.outer", "@loop.outer" },
|
|
i = { "@block.inner", "@conditional.inner", "@loop.inner" },
|
|
}),
|
|
f = ai.gen_spec.treesitter({ a = "@function.outer", i = "@function.inner" }), -- function
|
|
c = ai.gen_spec.treesitter({ a = "@class.outer", i = "@class.inner" }), -- class
|
|
t = { "<([%p%w]-)%f[^<%w][^<>]->.-</%1>", "^<.->().*()</[^/]->$" }, -- tags
|
|
d = { "%f[%d]%d+" }, -- digits
|
|
e = { -- Word with case
|
|
{ "%u[%l%d]+%f[^%l%d]", "%f[%S][%l%d]+%f[^%l%d]", "%f[%P][%l%d]+%f[^%l%d]", "^[%l%d]+%f[^%l%d]" },
|
|
"^().*()$",
|
|
},
|
|
i = LazyVim.mini.ai_indent, -- indent
|
|
g = LazyVim.mini.ai_buffer, -- buffer
|
|
u = ai.gen_spec.function_call(), -- u for "Usage"
|
|
U = ai.gen_spec.function_call({ name_pattern = "[%w_]" }), -- without dot in function name
|
|
},
|
|
}
|
|
end,
|
|
config = function(_, opts)
|
|
require("mini.ai").setup(opts)
|
|
LazyVim.on_load("which-key.nvim", function()
|
|
vim.schedule(function()
|
|
LazyVim.mini.ai_whichkey(opts)
|
|
end)
|
|
end)
|
|
end,
|
|
},
|
|
|
|
{
|
|
"folke/lazydev.nvim",
|
|
ft = "lua",
|
|
cmd = "LazyDev",
|
|
opts = {
|
|
library = {
|
|
{ path = "luvit-meta/library", words = { "vim%.uv" } },
|
|
{ path = "LazyVim", words = { "LazyVim" } },
|
|
{ path = "snacks.nvim", words = { "Snacks" } },
|
|
{ path = "lazy.nvim", words = { "LazyVim" } },
|
|
},
|
|
},
|
|
},
|
|
-- Manage libuv types with lazy. Plugin will never be loaded
|
|
{ "Bilal2453/luvit-meta", lazy = true },
|
|
-- Add lazydev source to cmp
|
|
{
|
|
"hrsh7th/nvim-cmp",
|
|
optional = true,
|
|
opts = function(_, opts)
|
|
table.insert(opts.sources, { name = "lazydev", group_index = 0 })
|
|
end,
|
|
},
|
|
}
|