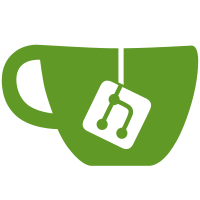
This patch makes the `make docs` directive incremental avoiding re-running the siphon when the source hasn't changed, and leveraging sphinx internal cache. It adds a `make rebuild-docs` directive for cases where this caching logic might break, e.g. in CI. The virtualenv doesn't also get recreated on each build, which might be enough when writing docs, provided automated process leverage its rebuild counterpart. Type: improvement Change-Id: Ie90de3adebeed017b249cad81c6c160719f71e8d Signed-off-by: Nathan Skrzypczak <nathan.skrzypczak@gmail.com> Signed-off-by: Dave Wallace <dwallacelf@gmail.com>
91 lines
3.3 KiB
Python
Executable File
91 lines
3.3 KiB
Python
Executable File
#!/usr/bin/env python3
|
|
# Copyright (c) 2016 Comcast Cable Communications Management, LLC.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License");
|
|
# you may not use this file except in compliance with the License.
|
|
# You may obtain a copy of the License at:
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS,
|
|
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
# See the License for the specific language governing permissions and
|
|
# limitations under the License.
|
|
|
|
# Filter for .siphon files that are generated by other filters.
|
|
# The idea is to siphon off certain initializers so that we can better
|
|
# auto-document the contents of that initializer.
|
|
|
|
import argparse
|
|
import logging
|
|
import os
|
|
import sys
|
|
|
|
import siphon
|
|
|
|
DEFAULT_LOGFILE = None
|
|
DEFAULT_LOGLEVEL = "warning"
|
|
DEFAULT_SIPHON = "clicmd"
|
|
DEFAULT_FORMAT = "markdown"
|
|
DEFAULT_OUTPUT = None
|
|
DEFAULT_TEMPLATES = os.path.dirname(__file__) + "/siphon_templates"
|
|
DEFAULT_OUTPUT_DIR = os.path.dirname(__file__) + "/siphon_docs"
|
|
DEFAULT_REPO_LINK = "https://github.com/FDio/vpp/blob/master/"
|
|
|
|
ap = argparse.ArgumentParser()
|
|
ap.add_argument("--log-file", default=DEFAULT_LOGFILE,
|
|
help="Log file [%s]" % DEFAULT_LOGFILE)
|
|
ap.add_argument("--log-level", default=DEFAULT_LOGLEVEL,
|
|
choices=["debug", "info", "warning", "error", "critical"],
|
|
help="Logging level [%s]" % DEFAULT_LOGLEVEL)
|
|
|
|
ap.add_argument("--type", '-t', metavar="siphon_type", default=DEFAULT_SIPHON,
|
|
choices=siphon.process.siphons.keys(),
|
|
help="Siphon type to process [%s]" % DEFAULT_SIPHON)
|
|
ap.add_argument("--format", '-f', default=DEFAULT_FORMAT,
|
|
choices=siphon.process.formats.keys(),
|
|
help="Output format to generate [%s]" % DEFAULT_FORMAT)
|
|
ap.add_argument("--output", '-o', metavar="file", default=DEFAULT_OUTPUT,
|
|
help="Output file (uses stdout if not defined) [%s]" %
|
|
DEFAULT_OUTPUT)
|
|
ap.add_argument("--templates", metavar="directory", default=DEFAULT_TEMPLATES,
|
|
help="Path to render templates directory [%s]" %
|
|
DEFAULT_TEMPLATES)
|
|
ap.add_argument("--outdir", metavar="directory", default=DEFAULT_OUTPUT_DIR,
|
|
help="Path to output rendered parts [%s]" %
|
|
DEFAULT_OUTPUT_DIR)
|
|
ap.add_argument("--repolink", metavar="repolink", default=DEFAULT_REPO_LINK,
|
|
help="Link to public repository [%s]" %
|
|
DEFAULT_REPO_LINK)
|
|
ap.add_argument("input", nargs='+', metavar="input_file",
|
|
help="Input .siphon files")
|
|
args = ap.parse_args()
|
|
|
|
logging.basicConfig(filename=args.log_file,
|
|
level=getattr(logging, args.log_level.upper(), None))
|
|
log = logging.getLogger("siphon_process")
|
|
|
|
# Determine where to send the generated output
|
|
if args.output is None:
|
|
out = sys.stdout
|
|
else:
|
|
out = open(args.output, "w+")
|
|
|
|
# Get our processor
|
|
klass = siphon.process.siphons[args.type]
|
|
processor = klass(
|
|
template_directory=args.templates,
|
|
format=args.format,
|
|
outdir=args.outdir,
|
|
repository_link=args.repolink
|
|
)
|
|
|
|
# Load the input files
|
|
processor.load_json(args.input)
|
|
|
|
# Process the data
|
|
processor.process(out=out)
|
|
|
|
# All done
|