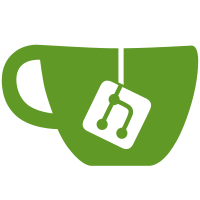
- Make framework.py classes a subset of asfframework.py classes - Remove all packet related code from asfframework.py - Add test class and test case set up debug output to log - Repatriate packet tests from asf to test directory - Remove non-packet related code from framework.py and inherit them from asfframework.py classes - Clean up unused import variables - Re-enable BFD tests on Ubuntu 22.04 and fix intermittent test failures in echo_looped_back testcases (where # control packets verified but not guaranteed to be received during test) - Re-enable Wireguard tests on Ubuntu 22.04 and fix intermittent test failures in handshake ratelimiting testcases and event testcase - Run Wiregard testcase suites solo - Improve debug output in log.txt - Increase VCL/LDP post sleep timeout to allow iperf server to finish cleanly. - Fix pcap history files to be sorted by suite and testcase and ensure order/timestamp is correct based on creation in the testcase. - Decode pcap files for each suite and testcase for all errors or if configured via comandline option / env var - Improve vpp corefile detection to allow complete corefile generation - Disable vm vpp interfaces testcases on debian11 - Clean up failed unittest dir when retrying failed testcases and unify testname directory and failed linknames into framwork functions Type: test Change-Id: I0764f79ea5bb639d278bf635ed2408d4d5220e1e Signed-off-by: Dave Wallace <dwallacelf@gmail.com>
59 lines
1.5 KiB
Python
59 lines
1.5 KiB
Python
from vpp_interface import VppInterface
|
|
|
|
|
|
class VppBondInterface(VppInterface):
|
|
"""VPP bond interface."""
|
|
|
|
def __init__(
|
|
self,
|
|
test,
|
|
mode,
|
|
lb=0,
|
|
numa_only=0,
|
|
enable_gso=0,
|
|
use_custom_mac=0,
|
|
mac_address="",
|
|
id=0xFFFFFFFF,
|
|
):
|
|
"""Create VPP Bond interface"""
|
|
super(VppBondInterface, self).__init__(test)
|
|
self.mode = mode
|
|
self.lb = lb
|
|
self.numa_only = numa_only
|
|
self.enable_gso = enable_gso
|
|
self.use_custom_mac = use_custom_mac
|
|
self.mac_address = mac_address
|
|
self.id = id
|
|
|
|
def add_vpp_config(self):
|
|
r = self.test.vapi.bond_create2(
|
|
self.mode,
|
|
self.lb,
|
|
self.numa_only,
|
|
self.enable_gso,
|
|
self.use_custom_mac,
|
|
self.mac_address,
|
|
self.id,
|
|
)
|
|
self.set_sw_if_index(r.sw_if_index)
|
|
|
|
def remove_vpp_config(self):
|
|
self.test.vapi.bond_delete(self.sw_if_index)
|
|
|
|
def add_member_vpp_bond_interface(
|
|
self, sw_if_index, is_passive=0, is_long_timeout=0
|
|
):
|
|
self.test.vapi.bond_add_member(
|
|
sw_if_index, self.sw_if_index, is_passive, is_long_timeout
|
|
)
|
|
|
|
def detach_vpp_bond_interface(self, sw_if_index):
|
|
self.test.vapi.bond_detach_member(sw_if_index)
|
|
|
|
def is_interface_config_in_dump(self, dump):
|
|
for i in dump:
|
|
if i.sw_if_index == self.sw_if_index:
|
|
return True
|
|
else:
|
|
return False
|