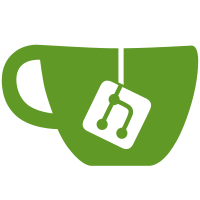
This module implement Python access to the VPP statistics segment. It accesses the data structures directly in shared memory. VPP uses optimistic locking, so data structures may change underneath us while we are reading. Data is copied out and it's important to spend as little time as possible "holding the lock". Counters are stored in VPP as a two dimensional array. Index by thread and index (typically sw_if_index). Simple counters count only packets, Combined counters count packets and octets. Counters can be accessed in either dimension. stat['/if/rx'] - returns 2D lists stat['/if/rx'][0] - returns counters for all interfaces for thread 0 stat['/if/rx'][0][1] - returns counter for interface 1 on thread 0 stat['/if/rx'][0][1]['packets'] - returns the packet counter for interface 1 on thread 0 stat['/if/rx'][:, 1] - returns the counters for interface 1 on all threads stat['/if/rx'][:, 1].packets() - returns the packet counters for interface 1 on all threads stat['/if/rx'][:, 1].sum_packets() - returns the sum of packet counters for interface 1 on all threads stat['/if/rx-miss'][:, 1].sum() - returns the sum of packet counters for interface 1 on all threads for simple counters Type: refactor Signed-off-by: Ole Troan <ot@cisco.com> Change-Id: I1fe7f7c7d11378d06be8276db5e1900ecdb8f515 Signed-off-by: Ole Troan <ot@cisco.com>
88 lines
2.5 KiB
Python
88 lines
2.5 KiB
Python
"""
|
|
Neighbour Entries
|
|
|
|
object abstractions for ARP and ND
|
|
"""
|
|
|
|
from ipaddress import ip_address
|
|
from vpp_object import VppObject
|
|
from vpp_papi import mac_pton, VppEnum
|
|
try:
|
|
text_type = unicode
|
|
except NameError:
|
|
text_type = str
|
|
|
|
|
|
def find_nbr(test, sw_if_index, nbr_addr, is_static=0, mac=None):
|
|
ip_addr = ip_address(text_type(nbr_addr))
|
|
e = VppEnum.vl_api_ip_neighbor_flags_t
|
|
nbrs = test.vapi.ip_neighbor_dump(sw_if_index=sw_if_index,
|
|
af=ip_addr.vapi_af)
|
|
|
|
for n in nbrs:
|
|
if sw_if_index == n.neighbor.sw_if_index and \
|
|
ip_addr == n.neighbor.ip_address and \
|
|
is_static == (n.neighbor.flags & e.IP_API_NEIGHBOR_FLAG_STATIC):
|
|
if mac:
|
|
if mac == str(n.neighbor.mac_address):
|
|
return True
|
|
else:
|
|
return True
|
|
return False
|
|
|
|
|
|
class VppNeighbor(VppObject):
|
|
"""
|
|
ARP Entry
|
|
"""
|
|
|
|
def __init__(self, test, sw_if_index, mac_addr, nbr_addr,
|
|
is_static=False, is_no_fib_entry=False):
|
|
self._test = test
|
|
self.sw_if_index = sw_if_index
|
|
self.mac_addr = mac_addr
|
|
self.nbr_addr = nbr_addr
|
|
|
|
e = VppEnum.vl_api_ip_neighbor_flags_t
|
|
self.flags = e.IP_API_NEIGHBOR_FLAG_NONE
|
|
if is_static:
|
|
self.flags |= e.IP_API_NEIGHBOR_FLAG_STATIC
|
|
if is_no_fib_entry:
|
|
self.flags |= e.IP_API_NEIGHBOR_FLAG_NO_FIB_ENTRY
|
|
|
|
def add_vpp_config(self):
|
|
r = self._test.vapi.ip_neighbor_add_del(
|
|
self.sw_if_index,
|
|
self.mac_addr,
|
|
self.nbr_addr,
|
|
is_add=1,
|
|
flags=self.flags)
|
|
self.stats_index = r.stats_index
|
|
self._test.registry.register(self, self._test.logger)
|
|
return self
|
|
|
|
def remove_vpp_config(self):
|
|
self._test.vapi.ip_neighbor_add_del(
|
|
self.sw_if_index,
|
|
self.mac_addr,
|
|
self.nbr_addr,
|
|
is_add=0,
|
|
flags=self.flags)
|
|
|
|
def is_static(self):
|
|
e = VppEnum.vl_api_ip_neighbor_flags_t
|
|
return (self.flags & e.IP_API_NEIGHBOR_FLAG_STATIC)
|
|
|
|
def query_vpp_config(self):
|
|
return find_nbr(self._test,
|
|
self.sw_if_index,
|
|
self.nbr_addr,
|
|
self.is_static())
|
|
|
|
def object_id(self):
|
|
return ("%d:%s" % (self.sw_if_index, self.nbr_addr))
|
|
|
|
def get_stats(self):
|
|
c = self._test.statistics["/net/adjacency"]
|
|
return c[0][self.stats_index]
|