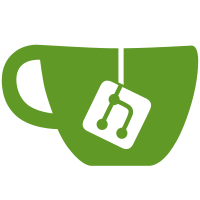
Exported indentifiers in Go start with capital letters. Only few fields in hs-test, which are being unmarshaled from yaml are required to be exported. Every other field name or method name should start with lower-case letter, to be consistent with this naming convention. Type: test Signed-off-by: Maros Ondrejicka <mondreji@cisco.com> Change-Id: I7eab0eef9fd08a7890c77b6ce1aeb3fa4b80f3cd
26 lines
597 B
Go
26 lines
597 B
Go
package main
|
|
|
|
import (
|
|
"fmt"
|
|
)
|
|
|
|
type NetDevConfig map[string]interface{}
|
|
type ContainerConfig map[string]interface{}
|
|
type VolumeConfig map[string]interface{}
|
|
|
|
type YamlTopology struct {
|
|
Devices []NetDevConfig `yaml:"devices"`
|
|
Containers []ContainerConfig `yaml:"containers"`
|
|
Volumes []VolumeConfig `yaml:"volumes"`
|
|
}
|
|
|
|
func addAddress(device, address, ns string) error {
|
|
c := []string{"ip", "addr", "add", address, "dev", device}
|
|
cmd := appendNetns(c, ns)
|
|
err := cmd.Run()
|
|
if err != nil {
|
|
return fmt.Errorf("failed to set ip address for %s: %v", device, err)
|
|
}
|
|
return nil
|
|
}
|