VPP-305: Documentation for vnet/vnet/unix
Change-Id: I3f1a225033ecebe0cedfc3466b552176461b76ab Signed-off-by: Keith Burns (alagalah) <alagalah@gmail.com>
This commit is contained in:
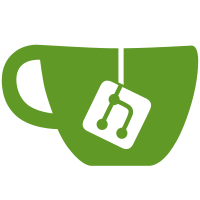
committed by
Damjan Marion
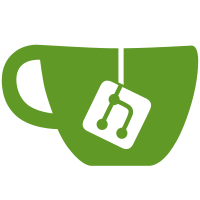
parent
c068179a6c
commit
07203afe6f
@ -12,33 +12,73 @@
|
||||
* See the License for the specific language governing permissions and
|
||||
* limitations under the License.
|
||||
*/
|
||||
/**
|
||||
* @file
|
||||
* @brief Host utility functions
|
||||
*/
|
||||
#include <vppinfra/format.h>
|
||||
#include <vlib/vlib.h>
|
||||
|
||||
#include <vlib/threads.h>
|
||||
|
||||
/* Functions to call from gdb */
|
||||
|
||||
|
||||
/**
|
||||
* @brief GDB callable function: vl - Return vector length of vector
|
||||
*
|
||||
* @param *p - void - address of vector
|
||||
*
|
||||
* @return length - u32
|
||||
*
|
||||
*/
|
||||
u32 vl(void *p)
|
||||
{
|
||||
return vec_len (p);
|
||||
}
|
||||
|
||||
/**
|
||||
* @brief GDB callable function: pe - call pool_elts - number of elements in a pool
|
||||
*
|
||||
* @param *v - void - address of pool
|
||||
*
|
||||
* @return number - uword
|
||||
*
|
||||
*/
|
||||
uword pe (void *v)
|
||||
{
|
||||
return (pool_elts(v));
|
||||
}
|
||||
|
||||
/**
|
||||
* @brief GDB callable function: pifi - call pool_is_free_index - is passed index free?
|
||||
*
|
||||
* @param *p - void - address of pool
|
||||
* @param *index - u32
|
||||
*
|
||||
* @return 0|1 - int
|
||||
*
|
||||
*/
|
||||
int pifi (void *p, u32 index)
|
||||
{
|
||||
return pool_is_free_index (p, index);
|
||||
}
|
||||
|
||||
/**
|
||||
* @brief GDB callable function: debug_hex_bytes - return formatted hex string
|
||||
*
|
||||
* @param *s - u8
|
||||
* @param n - u32 - number of bytes to format
|
||||
*
|
||||
*/
|
||||
void debug_hex_bytes (u8 *s, u32 n)
|
||||
{
|
||||
fformat (stderr, "%U\n", format_hex_bytes, s, n);
|
||||
}
|
||||
|
||||
/**
|
||||
* @brief GDB callable function: vlib_dump_frame_ownership
|
||||
*
|
||||
*/
|
||||
void vlib_dump_frame_ownership (void)
|
||||
{
|
||||
vlib_main_t * vm = vlib_get_main();
|
||||
@ -47,7 +87,7 @@ void vlib_dump_frame_ownership (void)
|
||||
vlib_next_frame_t * nf;
|
||||
u32 first_nf_index;
|
||||
u32 index;
|
||||
|
||||
|
||||
vec_foreach(this_node_runtime, nm->nodes_by_type[VLIB_NODE_TYPE_INTERNAL])
|
||||
{
|
||||
first_nf_index = this_node_runtime->next_frame_index;
|
||||
@ -74,11 +114,18 @@ void vlib_dump_frame_ownership (void)
|
||||
}
|
||||
}
|
||||
|
||||
/**
|
||||
* @brief GDB callable function: vlib_runtime_index_to_node_name
|
||||
*
|
||||
* Takes node index and will return the node name.
|
||||
*
|
||||
* @param index - u32
|
||||
*/
|
||||
void vlib_runtime_index_to_node_name (u32 index)
|
||||
{
|
||||
vlib_main_t * vm = vlib_get_main();
|
||||
vlib_node_main_t * nm = &vm->node_main;
|
||||
|
||||
|
||||
if (index > vec_len (nm->nodes))
|
||||
{
|
||||
fformat(stderr, "%d out of range, max %d\n", vec_len(nm->nodes));
|
||||
@ -89,6 +136,13 @@ void vlib_runtime_index_to_node_name (u32 index)
|
||||
}
|
||||
|
||||
|
||||
/**
|
||||
* @brief GDB callable function: show_gdb_command_fn - show gdb
|
||||
*
|
||||
* Shows list of functions for VPP available in GDB
|
||||
*
|
||||
* @return error - clib_error_t
|
||||
*/
|
||||
static clib_error_t *
|
||||
show_gdb_command_fn (vlib_main_t * vm,
|
||||
unformat_input_t * input,
|
||||
|
@ -40,24 +40,33 @@
|
||||
#include <vnet/unix/pcap.h>
|
||||
#include <sys/fcntl.h>
|
||||
|
||||
/* Usage
|
||||
|
||||
#include <vnet/unix/pcap.h>
|
||||
|
||||
static pcap_main_t pcap = {
|
||||
.file_name = "/tmp/ip4",
|
||||
.n_packets_to_capture = 2,
|
||||
.packet_type = PCAP_PACKET_TYPE_ip,
|
||||
};
|
||||
|
||||
To add a buffer:
|
||||
|
||||
pcap_add_buffer (&pcap, vm, pi0, 128);
|
||||
|
||||
file will be written after n_packets_to_capture or call to pcap_write (&pcap).
|
||||
|
||||
/**
|
||||
* @file
|
||||
* @brief PCAP function.
|
||||
* Usage
|
||||
*
|
||||
* #include <vnet/unix/pcap.h>
|
||||
*
|
||||
* static pcap_main_t pcap = {
|
||||
* .file_name = "/tmp/ip4",
|
||||
* .n_packets_to_capture = 2,
|
||||
* .packet_type = PCAP_PACKET_TYPE_ip,
|
||||
* };
|
||||
*
|
||||
* To add a buffer:
|
||||
*
|
||||
* pcap_add_buffer (&pcap, vm, pi0, 128);
|
||||
*
|
||||
* File will be written after n_packets_to_capture or call to pcap_write (&pcap).
|
||||
*
|
||||
*/
|
||||
|
||||
/**
|
||||
* @brief Close PCAP file
|
||||
*
|
||||
* @return rc - clib_error_t
|
||||
*
|
||||
*/
|
||||
clib_error_t *
|
||||
pcap_close (pcap_main_t * pm)
|
||||
{
|
||||
@ -67,6 +76,12 @@ pcap_close (pcap_main_t * pm)
|
||||
return 0;
|
||||
}
|
||||
|
||||
/**
|
||||
* @brief Write PCAP file
|
||||
*
|
||||
* @return rc - clib_error_t
|
||||
*
|
||||
*/
|
||||
clib_error_t *
|
||||
pcap_write (pcap_main_t * pm)
|
||||
{
|
||||
@ -143,6 +158,12 @@ pcap_write (pcap_main_t * pm)
|
||||
return error;
|
||||
}
|
||||
|
||||
/**
|
||||
* @brief Read PCAP file
|
||||
*
|
||||
* @return rc - clib_error_t
|
||||
*
|
||||
*/
|
||||
clib_error_t * pcap_read (pcap_main_t * pm)
|
||||
{
|
||||
clib_error_t * error = 0;
|
||||
@ -197,7 +218,7 @@ clib_error_t * pcap_read (pcap_main_t * pm)
|
||||
error = clib_error_return (0, "short read `%s'", pm->file_name);
|
||||
goto done;
|
||||
}
|
||||
|
||||
|
||||
if (vec_len (pm->packets_read) == 0)
|
||||
pm->min_packet_bytes = pm->max_packet_bytes = ph.n_bytes_in_packet;
|
||||
else
|
||||
@ -205,7 +226,7 @@ clib_error_t * pcap_read (pcap_main_t * pm)
|
||||
pm->min_packet_bytes = clib_min (pm->min_packet_bytes, ph.n_bytes_in_packet);
|
||||
pm->max_packet_bytes = clib_max (pm->max_packet_bytes, ph.n_bytes_in_packet);
|
||||
}
|
||||
|
||||
|
||||
vec_add1 (pm->packets_read, data);
|
||||
}
|
||||
|
||||
@ -213,5 +234,5 @@ clib_error_t * pcap_read (pcap_main_t * pm)
|
||||
if (fd >= 0)
|
||||
close (fd);
|
||||
return error;
|
||||
|
||||
|
||||
}
|
||||
|
@ -36,13 +36,25 @@
|
||||
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
||||
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
||||
*/
|
||||
|
||||
/**
|
||||
* @file
|
||||
* @brief PCAP utility definitions
|
||||
*/
|
||||
#ifndef included_vnet_pcap_h
|
||||
#define included_vnet_pcap_h
|
||||
|
||||
#include <vlib/vlib.h>
|
||||
|
||||
#define foreach_vnet_pcap_packet_type \
|
||||
/**
|
||||
* @brief Packet types supported by PCAP
|
||||
*
|
||||
* null 0
|
||||
* ethernet 1
|
||||
* ppp 9
|
||||
* ip 12
|
||||
* hdlc 104
|
||||
*/
|
||||
#define foreach_vnet_pcap_packet_type \
|
||||
_ (null, 0) \
|
||||
_ (ethernet, 1) \
|
||||
_ (ppp, 9) \
|
||||
@ -56,27 +68,27 @@ typedef enum {
|
||||
} pcap_packet_type_t;
|
||||
|
||||
#define foreach_pcap_file_header \
|
||||
/* 0xa1b2c3d4 host byte order. \
|
||||
/** 0xa1b2c3d4 host byte order. \
|
||||
0xd4c3b2a1 => need to byte swap everything. */ \
|
||||
_ (u32, magic) \
|
||||
\
|
||||
/* Currently major 2 minor 4. */ \
|
||||
/** Currently major 2 minor 4. */ \
|
||||
_ (u16, major_version) \
|
||||
_ (u16, minor_version) \
|
||||
\
|
||||
/* 0 for GMT. */ \
|
||||
/** 0 for GMT. */ \
|
||||
_ (u32, time_zone) \
|
||||
\
|
||||
/* Accuracy of timestamps. Typically set to 0. */ \
|
||||
/** Accuracy of timestamps. Typically set to 0. */ \
|
||||
_ (u32, sigfigs) \
|
||||
\
|
||||
/* Size of largest packet in file. */ \
|
||||
/** Size of largest packet in file. */ \
|
||||
_ (u32, max_packet_size_in_bytes) \
|
||||
\
|
||||
/* One of vnet_pcap_packet_type_t. */ \
|
||||
/** One of vnet_pcap_packet_type_t. */ \
|
||||
_ (u32, packet_type)
|
||||
|
||||
/* File header. */
|
||||
/** File header struct */
|
||||
typedef struct {
|
||||
#define _(t, f) t f;
|
||||
foreach_pcap_file_header
|
||||
@ -84,58 +96,79 @@ typedef struct {
|
||||
} pcap_file_header_t;
|
||||
|
||||
#define foreach_pcap_packet_header \
|
||||
/* Time stamp in seconds and microseconds. */ \
|
||||
/** Time stamp in seconds */ \
|
||||
_ (u32, time_in_sec) \
|
||||
/** Time stamp in microseconds. */ \
|
||||
_ (u32, time_in_usec) \
|
||||
\
|
||||
/* Number of bytes stored in file and size of actual packet. */ \
|
||||
/** Number of bytes stored in file. */ \
|
||||
_ (u32, n_packet_bytes_stored_in_file) \
|
||||
/** Number of bytes in actual packet. */ \
|
||||
_ (u32, n_bytes_in_packet)
|
||||
|
||||
/* Packet header. */
|
||||
/** Packet header. */
|
||||
typedef struct {
|
||||
#define _(t, f) t f;
|
||||
foreach_pcap_packet_header
|
||||
#undef _
|
||||
|
||||
/* Packet data follows. */
|
||||
/** Packet data follows. */
|
||||
u8 data[0];
|
||||
} pcap_packet_header_t;
|
||||
|
||||
/**
|
||||
* @brief PCAP main state data structure
|
||||
*/
|
||||
typedef struct {
|
||||
/* File name of pcap output. */
|
||||
/** File name of pcap output. */
|
||||
char * file_name;
|
||||
|
||||
/* Number of packets to capture. */
|
||||
/** Number of packets to capture. */
|
||||
u32 n_packets_to_capture;
|
||||
|
||||
/** Packet type */
|
||||
pcap_packet_type_t packet_type;
|
||||
|
||||
/* Number of packets currently captured. */
|
||||
/** Number of packets currently captured. */
|
||||
u32 n_packets_captured;
|
||||
|
||||
/** flags */
|
||||
u32 flags;
|
||||
#define PCAP_MAIN_INIT_DONE (1 << 0)
|
||||
|
||||
/* File descriptor for reading/writing. */
|
||||
/** File descriptor for reading/writing. */
|
||||
int file_descriptor;
|
||||
|
||||
/** Bytes written */
|
||||
u32 n_pcap_data_written;
|
||||
|
||||
/* Vector of pcap data. */
|
||||
/** Vector of pcap data. */
|
||||
u8 * pcap_data;
|
||||
|
||||
/* Packets read from file. */
|
||||
/** Packets read from file. */
|
||||
u8 ** packets_read;
|
||||
|
||||
/** Min/Max Packet bytes */
|
||||
u32 min_packet_bytes, max_packet_bytes;
|
||||
} pcap_main_t;
|
||||
|
||||
/* Write out data to output file. */
|
||||
/** Write out data to output file. */
|
||||
clib_error_t * pcap_write (pcap_main_t * pm);
|
||||
|
||||
/** Read data from file. */
|
||||
clib_error_t * pcap_read (pcap_main_t * pm);
|
||||
|
||||
/**
|
||||
* @brief Add packet
|
||||
*
|
||||
* @param *pm - pcap_main_t
|
||||
* @param time_now - f64
|
||||
* @param n_bytes_in_trace - u32
|
||||
* @param n_bytes_in_packet - u32
|
||||
*
|
||||
* @return Packet Data
|
||||
*
|
||||
*/
|
||||
static inline void *
|
||||
pcap_add_packet (pcap_main_t * pm,
|
||||
f64 time_now,
|
||||
@ -155,6 +188,15 @@ pcap_add_packet (pcap_main_t * pm,
|
||||
return h->data;
|
||||
}
|
||||
|
||||
/**
|
||||
* @brief Add buffer (vlib_buffer_t) to the trace
|
||||
*
|
||||
* @param *pm - pcap_main_t
|
||||
* @param *vm - vlib_main_t
|
||||
* @param buffer_index - u32
|
||||
* @param n_bytes_in_trace - u32
|
||||
*
|
||||
*/
|
||||
static inline void
|
||||
pcap_add_buffer (pcap_main_t * pm,
|
||||
vlib_main_t * vm, u32 buffer_index,
|
||||
@ -179,7 +221,7 @@ pcap_add_buffer (pcap_main_t * pm,
|
||||
b = vlib_get_buffer (vm, b->next_buffer);
|
||||
}
|
||||
|
||||
/* Flush output vector. */
|
||||
/** Flush output vector. */
|
||||
if (vec_len (pm->pcap_data) >= 64*1024
|
||||
|| pm->n_packets_captured >= pm->n_packets_to_capture)
|
||||
pcap_write (pm);
|
||||
|
@ -14,14 +14,21 @@
|
||||
* See the License for the specific language governing permissions and
|
||||
* limitations under the License.
|
||||
*/
|
||||
|
||||
/**
|
||||
* @file
|
||||
* @brief Functions to convert PCAP file format to VPP PG (Packet Generator)
|
||||
*
|
||||
*/
|
||||
#include <vnet/unix/pcap.h>
|
||||
#include <vnet/ethernet/packet.h>
|
||||
#include <stdio.h>
|
||||
|
||||
pcap_main_t pcap_main;
|
||||
|
||||
static char * pg_fmt =
|
||||
/**
|
||||
* @brief char * to seed a PG file
|
||||
*/
|
||||
static char * pg_fmt =
|
||||
"packet-generator new {\n"
|
||||
" name s%d\n"
|
||||
" limit 1\n"
|
||||
@ -29,16 +36,32 @@ static char * pg_fmt =
|
||||
" node ethernet-input\n";
|
||||
|
||||
|
||||
/**
|
||||
* @brief Packet Generator Stream boilerplate
|
||||
*
|
||||
* @param *ofp - FILE
|
||||
* @param i - int
|
||||
* @param *pkt - u8
|
||||
*/
|
||||
void stream_boilerplate (FILE *ofp, int i, u8 * pkt)
|
||||
{
|
||||
fformat(ofp, pg_fmt, i, vec_len(pkt), vec_len(pkt));
|
||||
}
|
||||
|
||||
/**
|
||||
* @brief Conversion of PCAP file to PG file format
|
||||
*
|
||||
* @param *pm - pcap_main_t
|
||||
* @param *ofp - FILE
|
||||
*
|
||||
* @return rc - int
|
||||
*
|
||||
*/
|
||||
int pcap2pg (pcap_main_t * pm, FILE *ofp)
|
||||
{
|
||||
int i, j;
|
||||
u8 *pkt;
|
||||
|
||||
|
||||
for (i = 0; i < vec_len (pm->packets_read); i++)
|
||||
{
|
||||
int offset;
|
||||
@ -51,13 +74,13 @@ int pcap2pg (pcap_main_t * pm, FILE *ofp)
|
||||
stream_boilerplate (ofp, i, pkt);
|
||||
|
||||
fformat (ofp, " data {\n");
|
||||
|
||||
|
||||
ethertype = clib_net_to_host_u16 (h->type);
|
||||
|
||||
/*
|
||||
/**
|
||||
* In vnet terms, packet generator interfaces are not ethernets.
|
||||
* They don't have vlan tables.
|
||||
* This dance transforms captured 802.1q VLAN packets into
|
||||
* This transforms captured 802.1q VLAN packets into
|
||||
* regular Ethernet packets.
|
||||
*/
|
||||
if (ethertype == 0x8100 /* 802.1q vlan */)
|
||||
@ -69,7 +92,7 @@ int pcap2pg (pcap_main_t * pm, FILE *ofp)
|
||||
else
|
||||
offset = 14;
|
||||
|
||||
fformat (ofp,
|
||||
fformat (ofp,
|
||||
" 0x%04x: %02x%02x.%02x%02x.%02x%02x"
|
||||
" -> %02x%02x.%02x%02x.%02x%02x\n",
|
||||
ethertype,
|
||||
@ -97,6 +120,10 @@ int pcap2pg (pcap_main_t * pm, FILE *ofp)
|
||||
return 0;
|
||||
}
|
||||
|
||||
/**
|
||||
* @brief pcap2pg.
|
||||
* usage: pcap2pg -i <input-file> [-o <output-file>]
|
||||
*/
|
||||
int main (int argc, char **argv)
|
||||
{
|
||||
unformat_input_t input;
|
||||
|
File diff suppressed because it is too large
Load Diff
@ -14,11 +14,16 @@
|
||||
* See the License for the specific language governing permissions and
|
||||
* limitations under the License.
|
||||
*/
|
||||
/**
|
||||
* @file
|
||||
* @brief TAPCLI definitions
|
||||
*/
|
||||
|
||||
#ifndef __included_tapcli_h__
|
||||
#define __included_tapcli_h__
|
||||
|
||||
#define foreach_tapcli_error \
|
||||
/** TAP CLI errors */
|
||||
#define foreach_tapcli_error \
|
||||
/* Must be first. */ \
|
||||
_(NONE, "no error") \
|
||||
_(READ, "read error") \
|
||||
@ -32,6 +37,7 @@ typedef enum {
|
||||
TAPCLI_N_ERROR,
|
||||
} tapcli_error_t;
|
||||
|
||||
/** TAP CLI interface details struct */
|
||||
typedef struct {
|
||||
u32 sw_if_index;
|
||||
u8 dev_name[64];
|
||||
|
File diff suppressed because it is too large
Load Diff
@ -1,4 +1,4 @@
|
||||
/*
|
||||
/*
|
||||
*------------------------------------------------------------------
|
||||
* tuntap.h - kernel stack (reverse) punt/inject path
|
||||
*
|
||||
@ -16,10 +16,9 @@
|
||||
* limitations under the License.
|
||||
*------------------------------------------------------------------
|
||||
*/
|
||||
|
||||
/*
|
||||
* Call from some VLIB_INIT_FUNCTION to set the Linux kernel
|
||||
* inject node name.
|
||||
/**
|
||||
* @file
|
||||
* @brief Call from VLIB_INIT_FUNCTION to set the Linux kernel inject node name.
|
||||
*/
|
||||
void register_tuntap_inject_node_name (char *name);
|
||||
|
||||
|
Reference in New Issue
Block a user